Xcode can compile C code without additional settings. Just add your C files to your project and select a proper target.
One way to use C code from Swift is with a bridging header (another way is to use a modulemap file, it's more flexible and convenient but also a bit more complex to setup).
Here is an example on how to use C and Swift in the same project, you can use the same technique with your existing C code.
Create a new iOS project.
Add a new C file with a corresponding header (Xcode will prompt you).

Xcode should suggest to add a bridging header and create it automatically. If it doesn't happen you can create it manually and add it to your project's Build Settings.
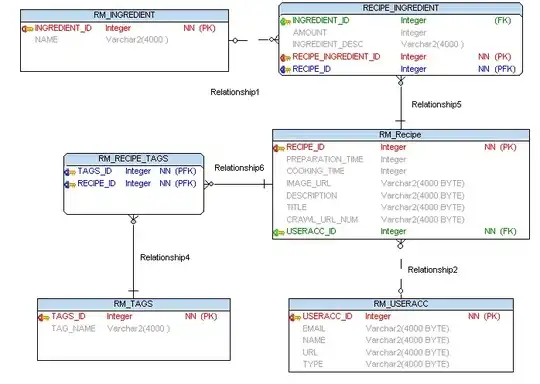
Add some code to your .c and .h files, for example:
// example.h
#include <stdio.h>
int exampleMax(int num1, int num2);
// example.c
#include "example.h"
int exampleMax(int num1, int num2)
{
int result;
if (num1 > num2)
result = num1;
else
result = num2;
return result;
}
Add your C header to the bridging file:
// ModuleName-Bridging-Header.h
#import "example.h"
Now you can call your C function from Swift:
import UIKit
class ViewController: UIViewController {
override func viewDidLoad() {
super.viewDidLoad()
let number1 = Int32(1)
let number2 = Int32(5)
let result = exampleMax(number1, number2)
print("min = \(result)")
}
}
Auto-completion should recognize your C interface:
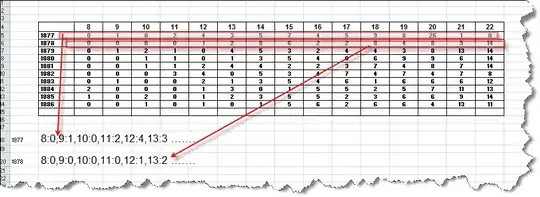