The same question also came to mind and finally, I found out why we can not override the prototype of the class, here is my answer:
See, prototype is a property of function /class which is an object, and if you examine the prototype object with Object.getOwnPropertyDescriptor(<your_class_or_function_name>,'prototype') ,in case of class you will find that "writable:false" but in case function "writable: true"
find below the code snippet :
In Case of Class
class myclass {
constructor(name) {
this.name = name;
}
getName() {
console.log(this.name);
}
}
console.log(Object.getOwnPropertyDescriptor(myclass, "prototype"));
Output:
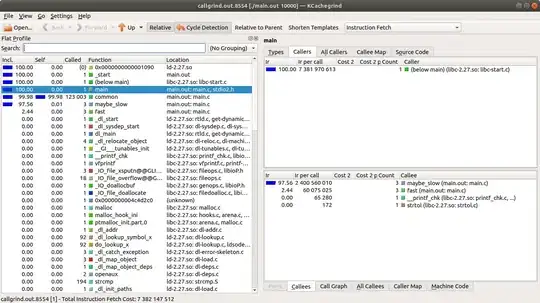
In Case of function:
function MyFunction(name) {
this.name = name;
}
console.log(Object.getOwnPropertyDescriptor(MyFunction, "prototype"));
Output:
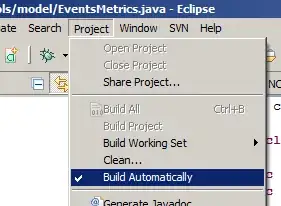
Hope you got the answer