Question 3 - text colour
how can i change the text color?
Solution 3 - Label color
Use color
to change the text colour
Note
The Button is a Label with associated actions that are
triggered when the button is pressed (or released after a
click/touch). To configure the button, the same properties (padding,
font_size, etc) and sizing system are used as for the Label class.
color
Text color, in the format (r, g, b, a).
color is a ListProperty and defaults to [1, 1, 1, 1].
Snippets
def apply_selection(self, rv, index, is_selected):
''' Respond to the selection of items in the view. '''
self.selected = is_selected
if rv.data[index]['text'].lower() == 'kyle':
self.color = [0, 1, 0, 1] # green colour text
...
elif rv.data[index]['text'].lower() == 'raul':
self.color = [0, 1, 1, 1] # aqua colour text
...
else:
self.color = [1, 1, 1, 1] # default white colour text
...
Question 1 - resize cell based on content
how can i fit the content within the cell or resize the cell based on the content size.
Solution 1 - Label texture_size
To set the size to the text content, bind size
to texture_size
to grow with the text.
texture_size
Texture size of the text. The size is determined by the font size and
text. If text_size is [None, None], the texture will be the size
required to fit the text, otherwise it’s clipped to fit
text_size.
When text_size is [None, None], one can bind to texture_size and
rescale it proportionally to fit the size of the label in order to
make the text fit maximally in the label.
Warning
The texture_size is set after the texture property. If you listen
for changes to texture, texture_size will not be up-to-date in
your callback. Bind to texture_size instead.
Snippets
<Label>:
size: self.texture_size
<TextInputPopup>:
title: "Popup"
Question 2 - color cell according to data
how can i change the color of cell based on the values in it
Solution 2
The solution requires changes to the Python script.
Py file
- Check for key e.g. kyle or raul in the method
apply_selection(self, rv, index, is_selected)
and set the rgba to red or blue respectively.
- Change the colour by using Button's attributes,
background_color
and background_normal
together.
background_color
Background color, in the format (r, g, b, a).
This acts as a multiplier to the texture colour. The default texture
is grey, so just setting the background color will give a darker
result. To set a plain color, set the background_normal to ''.
The background_color is a ListProperty and defaults to [1, 1, 1,
1].
background_normal
Background image of the button used for the default graphical
representation when the button is not pressed.
background_normal is a StringProperty and defaults to
‘atlas://data/images/defaulttheme/button’.
Snippets
def apply_selection(self, rv, index, is_selected):
''' Respond to the selection of items in the view. '''
self.selected = is_selected
if rv.data[index]['text'].lower() == 'kyle':
self.background_color = [1, 0, 0, 1] # red background colour
self.background_normal = ''
elif rv.data[index]['text'].lower() == 'raul':
self.background_color = [0, 0, 1, 1] # blue background colour
self.background_normal = ''
else:
self.background_color = [1, 1, 1, 1] # default colour
self.background_normal = 'atlas://data/images/defaulttheme/button' # default
Output
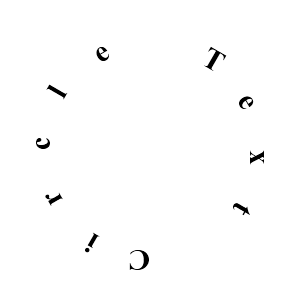