It may not be the best idea to do that with regular expressions. However, if you have to, you might want to create three capturing groups with parent open/close tags as left/right boundaries and swipe everything in between:
(<TopicSet.*?>)([\s\S]*?)(<\/TopicSet>)

RegEx
If this wasn't your desired expression, you can modify/change your expressions in regex101.com.
RegEx Circuit
You can also visualize your expressions in jex.im:
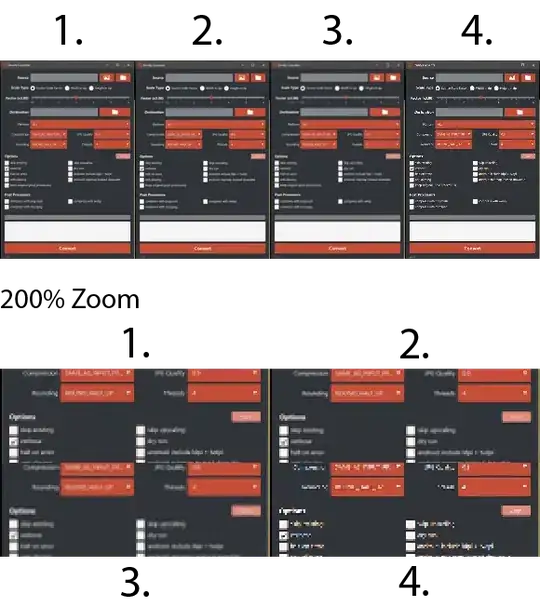
JavaScript Demo
const regex = /(<TopicSet.*?>)([\s\S]*?)(<\/TopicSet>)/mg;
const str = `<TopicSet FormalName="PARENT1">
<Topic>
<TopicType FormalName="Child1" />
</Topic>
<Topic>
<TopicType FormalName="Child2" />
</Topic>
<Topic>
<TopicType FormalName="Child3" />
</Topic>
</TopicSet>
<TopicSet FormalName="PARENT2">
<Topic>
<TopicType FormalName="Child1" />
</Topic>
<Topic>
<TopicType FormalName="Child2" />
</Topic>
<Topic>
<TopicType FormalName="Child3" />
</Topic>
</TopicSet>`;
const subst = `$2`;
// The substituted value will be contained in the result variable
const result = str.replace(regex, subst);
console.log('Substitution result: ', result);
JavaScript Demo 2
If you wish to also print the parent tag, you can simply replace it with $1$2$3
instead of $2
, which here we have added to be just simple to call:
const regex = /(<TopicSet.*?>)([\s\S]*?)(<\/TopicSet>)/mg;
const str = `<TopicSet FormalName="PARENT1">
<Topic>
<TopicType FormalName="Child1" />
</Topic>
<Topic>
<TopicType FormalName="Child2" />
</Topic>
<Topic>
<TopicType FormalName="Child3" />
</Topic>
</TopicSet>
<TopicSet FormalName="PARENT2">
<Topic>
<TopicType FormalName="Child1" />
</Topic>
<Topic>
<TopicType FormalName="Child2" />
</Topic>
<Topic>
<TopicType FormalName="Child3" />
</Topic>
</TopicSet>`;
const subst = `$1$2$3`;
// The substituted value will be contained in the result variable
const result = str.replace(regex, subst);
console.log('Substitution result: ', result);
If you only want to extract the first parent, you can add another boundary:
(<TopicSet FormalName="PARENT1">)([\s\S]*?)(<\/TopicSet>)