Spring security supports more-than-one roles out-of-the-box!
So, to save all of you fine folks a ton of time:
One must insert multiple entries for the same user:
That was in MySQL Workbench, with MySQL 5.7.24
Also other environments - in case you wondering which version to reproduce that result:
<!-- Inherit defaults from Spring Boot -->
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.1.1.RELEASE</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!-- optional, it brings useful tags to display spring security stuff -->
<dependency>
<groupId>org.thymeleaf.extras</groupId>
<artifactId>thymeleaf-extras-springsecurity5</artifactId>
</dependency>
Then to verify I made this page and:
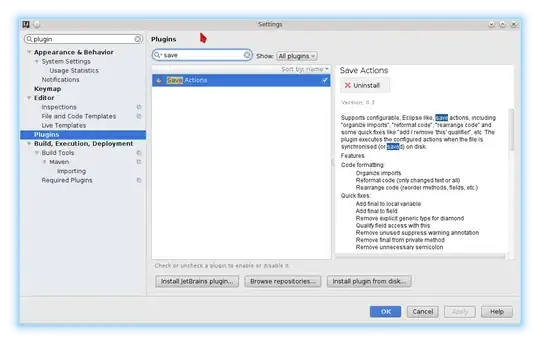
Here's the example code for displaying and verifying the logged in account's authorities:
<div data-layout-fragment="content" class="content">
<div class="row mt-4">
<div class="col-md-12">
<h2>Show Authorities Glance</h2>
<div class="card">
<div class="card-body">
Logged user: <span data-sec-authentication="name">Bob</span>
Roles: <span data-sec-authentication="principal.authorities">[ROLE_USER, ROLE_ADMIN]</span>
<div data-sec-authorize="isAuthenticated()">
This content is only shown to authenticated users.
</div>
<div data-sec-authorize="hasRole('ROLE_USER')">
This content is only shown to ROLE_USER.
</div>
<div data-sec-authorize="hasRole('ROLE_EMPLOYEE')">
This content is only shown to ROLE_EMPLOYEE.
</div>
<div data-sec-authorize="hasRole('ROLE_FOUNDER')">
This content is only shown to ROLE_FOUNDER.
</div>
<div data-sec-authorize="hasRole('ROLE_ADMIN')">
This content is only shown to ROLE_ADMIN.
</div>
</div>
</div>
</div>
</div>
</div>
<!--<p>-->
<!--<a data-th-href="@{/add-authority}">Add a new authority</a>-->
<!--</p>-->
</div>
Oh and this last view contains thymeleaf and not only the standard one also the layout dialect. Just in case you would like to try it out also need this dependency:
<dependency>
<groupId>nz.net.ultraq.thymeleaf</groupId>
<artifactId>thymeleaf-layout-dialect</artifactId>
</dependency>
Or get ride of the layout fragment tag:
data-layout-fragment="content"