I don't really perceive this as a tracking problem because the wheel is constrained so it can't move about all over the frame, it can only change its angular position, so you only really need to know where some part of it is in one frame and how much it has rotated by in the next frame. Then, as you know the framerate, i.e. the time between frames, you can calculate the speed.
So, the question is how to tell which is the same spoke that you measured in the previous frame. As the area behind the spokes is dark, you would want a light spoke to contrast well so you can find it easily. So, I would paint four of the spokes black, then you are just looking for one light one on a dark background. I would also consider painting the centre of the wheel red (or other saturated colour), so you can easily find the middle.
Now, at the start of processing, find the centre of the wheel by looking for red and get its x,y coordinates in the image. Now choose a radius in pixels that you can alter later, and work out a list of the x,y coordinates of say 360 points (1 per degree) on the circumference of the circle centred on and going around the red point. These points and all the sines/cosines will not change all through your processing, so do this outside your main video processing loop.
Now at each frame, use indexing to pick up the brightness at each of the 360 points and, initially at least, take the brightest one as the spoke.
So, I have crudely painted on your image so the centre is red and just one spoke is white:
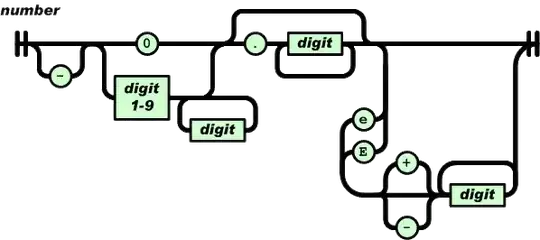
Now the code looks something like this:
#!/usr/bin/env python3
import math
import numpy as np
from PIL import Image
# Open image and make Numpy version of it too
im = Image.open('wheel.png')
imnp = np.array(im)
# Find centre by looking for red pixels
# See https://stackoverflow.com/a/52183666/2836621
x, y = 193, 168
# Set up list of 360 points on a circle centred on red dot outside main processing loop
radius = 60
# List of X values and Y values on circumference
Xs = []
Ys = []
for theta in range(360):
thetaRad = math.radians(theta)
dx = int(radius * math.sin(thetaRad))
dy = int(radius * math.cos(thetaRad))
Xs.append(x+dx)
Ys.append(y+dy)
# Your main loop processing frames starts here
# Make greyscale Numpy version of image
grey = np.array(im.convert('L'))
sum = 0
Bmax = 0
Tmax = 0
for theta in range(360):
brightness=grey[Ys[theta],Xs[theta]]
sum += brightness
if brightness > Bmax:
Bmax = brightness
Tmax = theta
print(f"theta: {theta}: brightness={brightness}")
# Calculate mean
Mgrey = sum/len(Xs)
print(f"Mean brightness on circumf: {Mgrey}")
# Print peak brightness and matching theta
print(f"Peak brightness: {Bmax} at theta: {Tmax}")
And the output is like this:
theta: 0: brightness=38
theta: 5: brightness=38
theta: 10: brightness=38
theta: 15: brightness=38
theta: 20: brightness=38
theta: 25: brightness=38
theta: 30: brightness=38
theta: 35: brightness=45
theta: 40: brightness=38
theta: 45: brightness=33
theta: 50: brightness=30
theta: 55: brightness=28
theta: 60: brightness=28
theta: 65: brightness=31
theta: 70: brightness=70
theta: 75: brightness=111
theta: 80: brightness=130
theta: 85: brightness=136
theta: 90: brightness=139 <--- peak brightness at 90 degrees to vertical as per picture - thankfully!
theta: 95: brightness=122
theta: 100: brightness=82
theta: 105: brightness=56
theta: 110: brightness=54
theta: 115: brightness=49
theta: 120: brightness=43
theta: 125: brightness=38
theta: 130: brightness=38
theta: 135: brightness=38
theta: 140: brightness=38
theta: 145: brightness=38
theta: 150: brightness=38
theta: 155: brightness=38
theta: 160: brightness=38
theta: 165: brightness=38
theta: 170: brightness=38
theta: 175: brightness=38
theta: 180: brightness=31
theta: 185: brightness=33
theta: 190: brightness=38
theta: 195: brightness=48
theta: 200: brightness=57
theta: 205: brightness=38
theta: 210: brightness=38
theta: 215: brightness=38
theta: 220: brightness=38
theta: 225: brightness=38
theta: 230: brightness=38
theta: 235: brightness=38
theta: 240: brightness=38
theta: 245: brightness=38
theta: 250: brightness=52
theta: 255: brightness=47
theta: 260: brightness=36
theta: 265: brightness=35
theta: 270: brightness=32
theta: 275: brightness=32
theta: 280: brightness=29
theta: 285: brightness=38
theta: 290: brightness=38
theta: 295: brightness=38
theta: 300: brightness=38
theta: 305: brightness=38
theta: 310: brightness=38
theta: 315: brightness=38
theta: 320: brightness=39
theta: 325: brightness=40
theta: 330: brightness=42
theta: 335: brightness=42
theta: 340: brightness=40
theta: 345: brightness=36
theta: 350: brightness=35
theta: 355: brightness=38
Mean brightness on circumf: 45.87222222222222
Peak brightness: 142 at theta: 89
If, in the next frame the peak brightness is now at 100 degrees to vertical, you know the wheel has rotated 10 degrees in 1/(frames_per_second).
You may need to vary the radius for best results - experiment! The white radius shown on the image corresponds to the 60 pixels radius in the code.
Rather than taking the peak brightness, you may want to find the mean and standard deviation of the brightness of the 360 pixels on the circumference and then take the angle as the average of the angles where the brightness is more than some number of standard deviations above the mean. It depends on the resolution/accuracy you need.
You can also collect all the brightnesses around the circle indexed by theta into a single 360-element array like this:
brightnessByTheta = grey[Ys[:],Xs[:]]
and you'll get:
array([ 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 43, 49, 47, 46, 45, 44, 43, 43,
40, 38, 36, 34, 33, 33, 33, 32, 31, 31, 29, 30, 28,
29, 29, 29, 28, 28, 27, 29, 28, 28, 27, 28, 28, 29,
31, 36, 42, 51, 60, 70, 81, 89, 98, 105, 111, 117, 122,
126, 128, 130, 131, 132, 133, 135, 136, 138, 139, 141, 142, 139,
136, 133, 129, 124, 122, 119, 113, 104, 93, 82, 72, 65, 60,
59, 56, 56, 55, 55, 54, 54, 53, 52, 52, 50, 49, 47,
46, 45, 44, 43, 42, 40, 39, 38, 38, 37, 38, 38, 37,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 34, 31, 31, 31, 31,
31, 31, 32, 33, 34, 35, 36, 37, 38, 42, 43, 44, 45,
48, 49, 50, 51, 55, 57, 60, 64, 65, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 52, 56, 46, 46, 47, 47, 38, 39, 40, 40,
36, 36, 36, 36, 36, 35, 35, 34, 34, 34, 32, 33, 33,
33, 33, 32, 32, 31, 30, 29, 29, 28, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38, 38,
38, 38, 38, 38, 38, 38, 40, 40, 39, 38, 39, 39, 39,
40, 40, 41, 41, 42, 42, 42, 41, 41, 42, 42, 41, 40,
39, 40, 40, 38, 39, 38, 37, 36, 36, 35, 34, 33, 35,
38, 38, 38, 38, 38, 38, 38, 38, 38], dtype=uint8)