As vahancho said in a comment, both odd[]
and even[]
are static arrays. That means once created you can't change the size of any of them. Moreover, when declaring the array, the size cannot be "dynamic", ie int odd[n];
is only valid when n
is a compile time constant (note that some compiler offer it as extension but it is not standard c++).
So here we get a bit stuck, and most would consider using the usual std::vector
which have a variable size.
Unfortunately, you specified "Use arrays" in your question. Erf, back to square one.
Let's think a little bit and remember that arrays can be seen as pointers. In the following image, we define an array arr[5]
. Then arr
is a pointer to the adress 1000, the first value of your array : 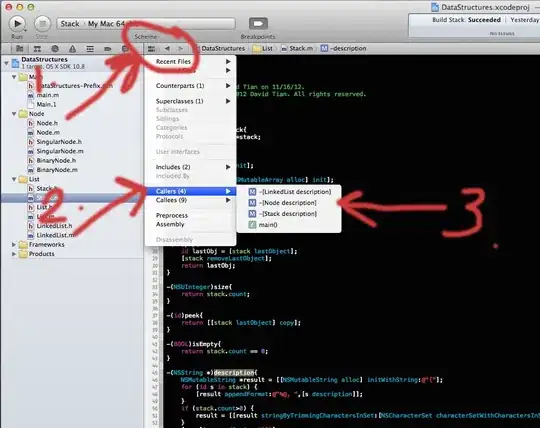
Spoiler alert : You can create a dynamic array with this property.
Ok, so let's define a pointer for our two arrays of odd and even numbers and let's declare their associated size:
int * odd_array = NULL;
int * even_array = NULL;
int odd_array_size = 0, even_array_size = 0;
Now, the idea is to increase the size of the good array when we find an odd or even number and use the C function void* realloc(void* ptr, size_t size)
offered by <cstdlib>
to increase the size allocated for your array. In your case you probably want to do that as you are looping over your input
array. Here is an exemple of what you can have in your case :
for (int n = 0; n < 10; n++)
{
if ((input_array[n]) % 2 != 0) // We find an odd number
{
odd_array_size++; // Increase the size of the array
odd_array = (int*)realloc(odd_array, odd_array_size * sizeof(int)); // Reallocate more memory
odd_array[odd_array_size-1] = input_array[n]; // Add the value in your new allocated memory
}
else
{
// Same here, but in the case of an even number
even_array_size++;
even_array = (int*)realloc(even_array, even_array_size * sizeof(int));
even_array[even_array_size-1] = input_array[n];
}
}
With this, you get your two arrays odd_array
and even_array
, filled respectively with the odd and even numbers of your input_array
, which both have their associated size odd_array_size
and even_array_size
This is basically a C way to do this. You may probably consider smart pointers (in order to be safe with freeing the memory) and, if you were allowed, std::vectors
which are the best way to deal with this problem. So don't forget to free your two arrays at the end of your program if you use this.
I hope this will be helpful and clear, don't hesitate to ask me clarifications in comment if needed.