What you're seeing is that R doesn't know how to guess an appropriate range for a single point. Typically it expands the range of data by 4% of the range (look at ?par
and look for 'xaxs'
), but with a single point that means nothing.
So we need to tell it what ylim
to use. (Similarly, your x-axis needs some guidance, too.)
Fake data:
set.seed(2)
coords <- data.frame(tm = Sys.time() + runif(20, -3e7, 3e7))
coords$month <- as.integer(format(coords$tm, format = "%m"))
coords$format_time <- format(coords$tm, format = "%l:%M:%S %p")
head(coords)
# tm month format_time
# 1 2018-10-24 20:15:17 10 8:15:17 PM
# 2 2019-10-19 05:07:04 10 5:07:04 AM
# 3 2019-07-21 14:19:22 7 2:19:22 PM
# 4 2018-10-13 03:44:57 10 3:44:57 AM
# 5 2020-04-03 21:32:22 4 9:32:22 PM
# 6 2020-04-03 15:27:59 4 3:27:59 PM
The "normal" plot looks fine:
month <- coords$month
time <- strptime(coords$format_time, format = "%l:%M:%S %p")
plot(month, time)
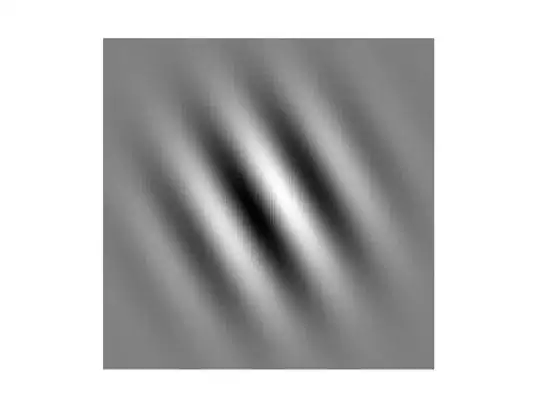
but the single-point does not:
sub <- coords[1,]
month <- sub$month
time <- strptime(sub$format_time, format = "%l:%M:%S %p")
plot(month, time)

So we fix it by specifying the xlim
and ylim
arguments. In this case, since I'm inferring it is meant to be a year of months (x) and a day of times (y), I can hard-code them, but in other situations you might want to just substract/add a small amount from the one datum you have:
sub <- coords[1,]
month <- sub$month
time <- strptime(sub$format_time, format = "%l:%M:%S %p")
xlim <- c(1, 12)
ylim <- strptime(c("12:00:00 AM", "11:59:59 PM"), format = "%l:%M:%S %p")
plot(month, time, xlim = xlim, ylim = as.numeric(ylim))
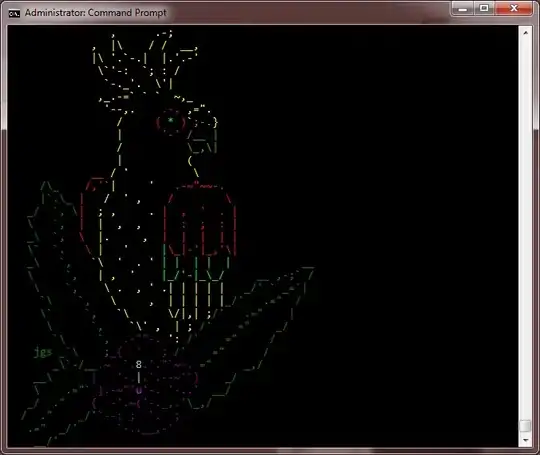
You only need to specify ylim
to answer this question, but without setting xlim=
here, the previous x-axis spanned 6-14, not good for months. Also of note is that I had to coerce ylim
to numeric for the plot, it did not work with ylim
in its pure POSIXt
form ... not sure exactly why that is the case, but this doesn't detract from the utility of the plot in general.