Its Seems you are trying to send POST
request to Azure Function V2
. See the below code snippet.
Custom Request Class:
public class Users
{
public string Name { get; set; }
public string Email { get; set; }
}
Azure Function V2:
In this example I am taking two parameter using a custom class and returned that two class property as response.
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
//Read Request Body
var content = await new StreamReader(req.Body).ReadToEndAsync();
//Extract Request Body and Parse To Class
Users objUsers = JsonConvert.DeserializeObject<Users>(content);
//As we have to return IAction Type So converting to IAction Class Using OkObjectResult We Even Can Use OkResult
var result = new OkObjectResult(objUsers);
return (IActionResult)result;
}
Request Sample:
{
"Name": "Kiron" ,
"Email": "kiron@email.com"
}
PostMan Test:
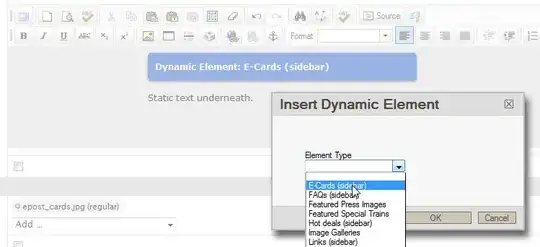
Note:
You are looking for await req.Content.ReadAsAsync<>();
this actually
needed for sending a POST
request from your function. And Read from
that server responses. But remember that req.Content
is not supported as reading post request in Azure Function V2
which have shown Function V1
example here
Another Example:
See the below code snippet:
public static async Task<IActionResult> Run(
[HttpTrigger(AuthorizationLevel.Anonymous, "get", "post", Route = null)] HttpRequest req,
ILogger log)
{
log.LogInformation("C# HTTP trigger function processed a request.");
//Read Request Body
var content = await new StreamReader(req.Body).ReadToEndAsync();
//Extract Request Body and Parse To Class
Users objUsers = JsonConvert.DeserializeObject<Users>(content);
//Post Reuqest to another API
HttpClient client = new HttpClient();
var json = JsonConvert.SerializeObject(objUsers);
//Parsing json to post request content
var stringContent = new StringContent(json, UnicodeEncoding.UTF8, "application/json");
//Posting data to remote API
HttpResponseMessage responseFromApi = await client.PostAsync("YourRequstURL", stringContent);
//Variable for next use to bind remote API response
var remoteApiResponse = "";
if (responseFromApi.IsSuccessStatusCode)
{
remoteApiResponse = responseFromApi.Content.ReadAsStringAsync().Result; // According to your sample, When you read from server response
}
//As we have to return IAction Type So converting to IAction Class Using OkObjectResult We Even Can Use OkResult
var result = new OkObjectResult(remoteApiResponse);
return (IActionResult)result;
}
Hope you understand, If you still have any query feel free to share. Thanks and happy coding!