This is a classic reason to use one list of similarly structured objects and not many separate objects, flooding your global environment and requiring extensive maintenance and search/recall. When objects are saved to a list you can run consistent operations on the elements like individual qplots or one large qplot.
Below are equivalent calls to save all data frames into a single, named list but as @Gregor advises: avoid having a bunch of data.frames not in a list ... don't wait until you have a bunch of a data.frames
to add them to a list. Start with the list.
df_list <- Filter(is.data.frame, eapply(.GlobalEnv, identity))
df_list <- Filter(is.data.frame, mget(x=ls(), envir=.GlobalEnv))
From there, use lapply
for individual plots or concatenate all data frames into a single data frame with indicator field and run facets.
# INDIVIDUAL PLOTS ------------------------------------------------
lapply(df_list, function(df) qplot(df$z, df$x, geom='smooth'))
# SINGLE PLOT -----------------------------------------------------
# ADD INDICATOR COLUMN IN EACH DF
df_list <- Map(function(df, nm) transform(df, a_name = nm), df_list, names(df_list))
# CONCATENATE ALL DF ELEMENTS
final_df <- do.call(rbind, unname(df_list))
# QPLOT WITH FACETS
with(final_df, qplot(z, x, geom='smooth', facets= ~ num))
To demonstrate with random, seeded data of nine data frames:
set.seed(662019)
a_names <- paste0("a", 1:9)
# BUILD DF LIST EACH WITH INDICATOR COLUMN
df_list <- lapply(a_names, function(i) data.frame(a_name = i, x = runif(100), z = runif(100)))
# CONCATENATE ALL DF ELEMENTS
final_df <- do.call(rbind, unname(df_list))
# QPLOT WITH FACETS
with(final_df, qplot(z, x, geom='smooth', facets= ~ a_name))
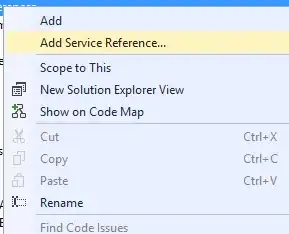