For my problem, I had a setup like this:
public var body: some View {
Form {
Text("Lorem ipsum dolor sit amet, consectetur adipiscing elit.")
.font(.title)
Spacer()
.fixedSize()
Text("""
Integer ut orci odio. Proin cursus ut elit eget rutrum. Nunc ante sem, euismod sed purus sed, tempus elementum elit. Phasellus lobortis at arcu quis porta. Cras accumsan leo eu tempus molestie. Suspendisse vulputate diam ipsum, et tristique lorem porta et. Pellentesque sodales est id arcu luctus venenatis.
Vestibulum non magna lorem. In tincidunt aliquet nunc, sit amet pharetra neque hendrerit id.
Cras sed!
""")
NativeButton("OK", keyEquivalent: .return) { self.screen = .game }
}
.frame(maxWidth: 480)
.fixedSize()
.padding()
}
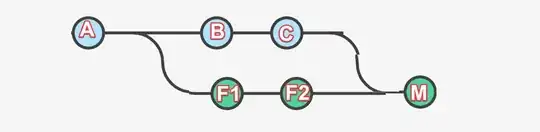
For some reason, all I had to do was add minWidth: 480, idealWidth: 480
to the frame and everything rendered correctly. I didn't expect this because I already applied .fixedSize()
, so I figured one of these three should've been enough.
public var body: some View {
Form {
Text("Lorem ipsum dolor sit amet, consectetur adipiscing elit.")
.font(.title)
Spacer()
.fixedSize()
Text("""
Integer ut orci odio. Proin cursus ut elit eget rutrum. Nunc ante sem, euismod sed purus sed, tempus elementum elit. Phasellus lobortis at arcu quis porta. Cras accumsan leo eu tempus molestie. Suspendisse vulputate diam ipsum, et tristique lorem porta et. Pellentesque sodales est id arcu luctus venenatis.
Vestibulum non magna lorem. In tincidunt aliquet nunc, sit amet pharetra neque hendrerit id.
Cras sed!
""")
NativeButton("OK", keyEquivalent: .return) { self.screen = .game }
}
.frame(minWidth: 480, idealWidth: 480, maxWidth: 480)
.fixedSize()
.padding()
}
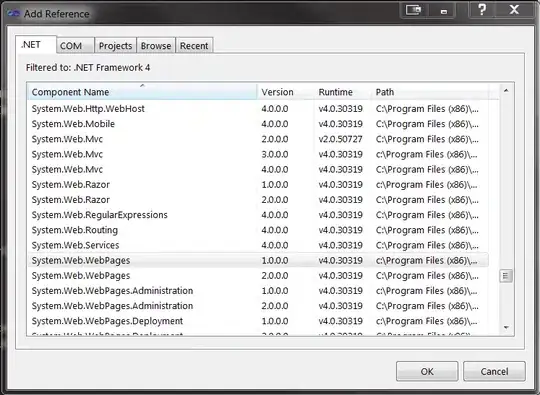