The OP wants to create a review matrix in which the names of the reviewed employees are chosen at random, the reviewer cannot review themselves, and the matrix is completed for 46 employees.
Based on previous code, this version builds an array of employee names for each row, in which the name of the reviewer is not included in the array. Five names are chosen at random and applied to the reviewer. The loop then repeats through each of the 46 employees.
For example, in the first round of reviews, "name01" is omitted from the array of employees from which the "reviewees" are randomly chosen. In the second round, "name01" is included, but "name02" is excluded from the array of employees. And so on, such that in each case, the array of employees used for the random selection of five reviews is always 45 names in length, and excludes the name of the reviewer.
The random selection of names to be rated does not ensure an equal and even distribution of reviews among employees. Though each employee will conduct 5 reviews, some employees are reviewed more than 5 times, some less than 5 times, and (depending on the alignment of the sun, the moon and the stars) it is possible that some may not be selected for review.
function s05648755803(){
var ss = SpreadsheetApp.getActiveSpreadsheet();
var sheetname = "Sheet3";
var sheet = ss.getSheetByName(sheetname);
// some variables
var randomcount = 30; // how many random names
var rowstart = 7; // ignore row 1 - the header row
var width = 5; // how many names in each row - 1/rater plus 5/ratee
var thelastrow = sheet.getLastRow();
//Logger.log("DEBUG:last row = "+thelastrow)
// get the employee names
var employeecount = thelastrow-rowstart+1;
//Logger.log("DEBUG: employee count = "+employeecount);//DEBUG
// get the data
var datarange = sheet.getRange(rowstart, 1, thelastrow - rowstart+1);
//Logger.log("DEBUG: range = "+datarange.getA1Notation());//DEBUG
var data = datarange.getValues();
//Logger.log("data length = "+data.length);
//Logger.log(data);
var counter = 0;
var newarray = [];
for (c = 0;c<46;c++){
counter = c;
for (i=0;i<data.length;i++){
if(i!=counter){
newarray.push(data[i]);
}
}
//Logger.log(newarray);
var rowdata = [];
var results = selectRandomElements(newarray, 5);
Logger.log(results)
rowdata.push(results);
var newrange = sheet.getRange(rowstart+c, 3, 1, 5);
newrange.setValues(rowdata);
// clear the arrays for the next loop
var newarray=[];
var rowdata = []
}
}
/*
// selectRandomElements and getRandomInt
// Credit: Vidar S. Ramdal
// https://webapps.stackexchange.com/a/102666/196152
*/
function selectRandomElements(fromValueRows, count) {
var pickedRows = []; // This will hold the selected rows
for (var i = 0; i < count && fromValueRows.length > 0; i++) {
var pickedIndex = getRandomInt(0, fromValueRows.length);
// Pick the element at position pickedIndex, and remove it from fromValueRows. See https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/splice
var pickedRow = fromValueRows.splice(pickedIndex, 1)[0];
// Add the selected row to our result array
pickedRows.push(pickedRow);
}
return pickedRows;
}
function getRandomInt(min,
max) { // From https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/random
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min)) + min;
}
Screenshot#1
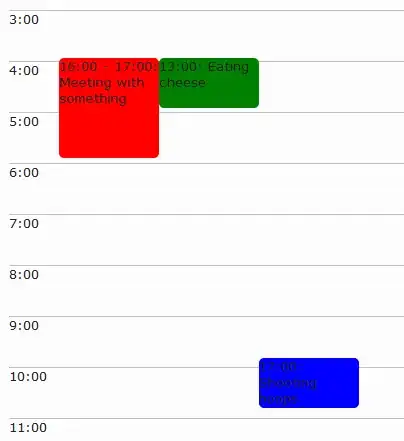
Screenshot#2
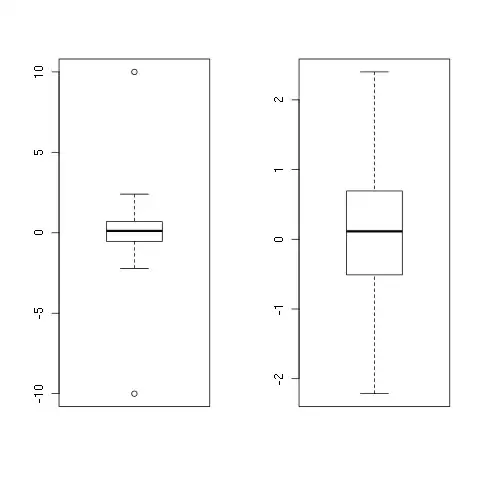