It's not going to make a difference you could detect, not even on a very, very large array. But if you're curious, you could always profile it on the JavaScript engines you care about and using your real code (since synthetic code gives synthetic results).
In specification terms, evaluating function code that completes with a return
(including an arrow's implicit return) vs. just "falling off" the end of the code results in two different kinds of completion, and then the process of calling that function differentiates between the return and the non-return and supplies undefined
in the non-return case. forEach
's code doesn't use the result, of course, but theoretically there could be different code paths within the JavaScript engine to get there, so theoretically they could have slightly different performance.
In practice, though, I think you can safely assume JavaScript engines optimize this quite well and the difference isn't apparent.
In general, don't optimize in advance. Respond to a performance problem in a given bit of code when you have a performance problem in a given bit of code. (That said, I totally understand being interested in this sort of thing in the abstract...)
Just for fun I did a jsPerf, don't see any sigificant difference in that synthetic test on Chrome (V8) or Firefox (SpiderMonkey):
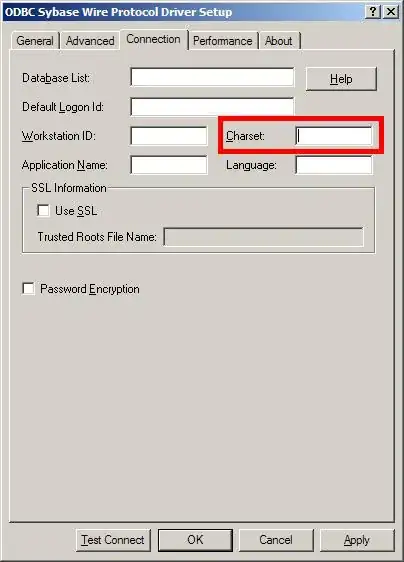
But again, synthetic tests give synthetic results.