Here's a basic card with Input.ChoiceSet:
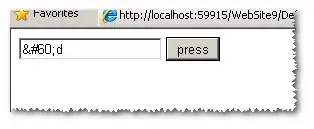
{
"type": "AdaptiveCard",
"body": [
{
"type": "Container",
"items": [
{
"type": "Input.ChoiceSet",
"id": "first",
"placeholder": "Placeholder text",
"choices": [
{
"title": "SharePoint",
"value": "SharePoint"
},
{
"title": "Azure",
"value": "Azure"
},
{
"title": "O365",
"value": "O365"
}
],
"style": "expanded"
}
]
}
],
"actions": [
{
"type": "Action.Submit",
"title": "Submit"
}
],
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"version": "1.0"
}
Then, in your bot, follow these answers for dealing with User Input:
Basically, you'll want to:
Send the card
Capture the card's input by sending a blank text prompt right after sending the card (as explained in the first link)
Use if or switch statements in the next step to determine which card to display next based off of the user's input. You could optionally create the card more dynamically using the second link
The AdaptiveCards Designer is pretty good, but it lacks the ability to set the actions
property. You can do so manually, by adding (like I did above):
"actions": [
{
"type": "Action.Submit",
"title": "Submit"
}
],
Using Images
If you'd like to use images instead of a ChoiceSet
, you can do something like this:
{
"type": "AdaptiveCard",
"body": [
{
"type": "Container",
"items": [
{
"type": "Image",
"id": "choice1",
"selectAction": {
"type": "Action.Submit",
"title": "choice1",
"data": {
"choice": 1
}
},
"url": "https://acuvate.com/wp-content/uploads/2017/02/Microsoft-Botframework.fw_-thegem-person.png",
"altText": ""
},
{
"type": "Image",
"id": "choice2",
"selectAction": {
"type": "Action.Submit",
"title": "choice2",
"data": {
"choice": 2
}
},
"url": "https://acuvate.com/wp-content/uploads/2017/02/Microsoft-Botframework.fw_-thegem-person.png",
"altText": ""
},
{
"type": "Image",
"id": "choice3",
"selectAction": {
"type": "Action.Submit",
"title": "choice3",
"data": {
"choice": 3
}
},
"url": "https://acuvate.com/wp-content/uploads/2017/02/Microsoft-Botframework.fw_-thegem-person.png",
"altText": ""
}
]
}
],
"$schema": "http://adaptivecards.io/schemas/adaptive-card.json",
"version": "1.0"
}
The important part is making sure that the Action.Submit
:
- Is on the image
- Has
data
that you would use to capture which choice the user selected