Personally, I am not a big fan absolute xpath. However, you can get the absolute xpath using javascript rather having the function in your language, which will ran faster and it's easy to port.
Here is the javascript.
// this function will return the absolute xpath of any given element
jsFunction = """window.getAbsoluteXpath =function(el){
// initialize the variables
aPath ="";
// iterate until the tag name is 'HTML'
while (el.tagName!='HTML'){
// get parent node
pEle=el.parentNode;
// check if there are more than 1 nodes with the same tagname under the parent
if(pEle.querySelectorAll(el.tagName).length>1){
//now findout the index of the current child
cIndex = 0;
pEle.querySelectorAll(el.tagName).forEach(function(cEle){
cIndex= cIndex+1;
// check if iterating ChildNode is equal to current ChildNode
if(cEle === el){
// set the aPath using index
aPath = el.tagName + "[" + cIndex + "]" + "/" +aPath;
}
})
}else{
// simply add the tagName when there is only one child with the tag name
aPath = el.tagName + "/" +aPath;
}
// set parent node as current element
el=el.parentNode;
}
// append HTML to the absolute xpath generated
return "//HTML/"+aPath.substring(0,aPath.length-1);
};"""
Now you can call this method in your javascript and pass element that you are interested in getting the absolute xpath.
Let's try to get the absolute xpath of
in stackoverflow.
Note: Did not tested the below code logic due to lack of environment on my machine.
# run the javascript in browser so that you can call the function anytime in your script
remDr %>% executeScript(jsFunction, args = list())
# get stackoverflow `Achievements` link element
webElem <- remDr %>% findElement("css", "a.-link.js-achievements-button")
# # get the absolute xpath of Stackoverflow `Achievements`
remDr %>% executeScript("return getAbsoluteXpath(arguments[0])", args = list(webElem))
Screenshot: Ran the javascript in chrome browser console for evidence
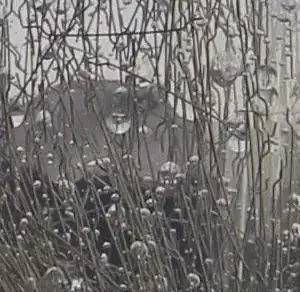