The torus is clipped by the near plane of the projection.
Change the projection matrix:
proj_matrix = m3dPerspective(m3dDegToRad(60.0), float(self.width) / float(self.height), 0.1, 100.0);
and the view matrix:
mv_matrix = (GLfloat * 16)(*identityMatrix)
m3dRotationMatrix44(mv_matrix, currentTime * m3dDegToRad(45.0), 1.0, 0.0, 0.0)
m3dTranslateMatrix44(mv_matrix, 0.0, 0.0, -3.0)
If you want to concatenate different matrices, then you've to implement an matrix "multiplication":
def m3dMultiply(A, B):
C = (GLfloat * 16)(*identityMatrix)
for k in range(0, 4):
for j in range(0, 4):
C[k*4+j] = A[0*4+j] * B[k*4+0] + A[1*4+j] * B[k*4+1] + \
A[2*4+j] * B[k*4+2] + A[3*4+j] * B[k*4+3]
return C
e.g. Concatenate a translation matrix and the rotation matrices around x and y axis:
T = (GLfloat * 16)(*identityMatrix)
m3dTranslateMatrix44(T, 0, 0, -4)
RX = (GLfloat * 16)(*identityMatrix)
m3dRotationMatrix44(RX, currentTime * m3dDegToRad(17.0), 1.0, 0.0, 0.0)
RY = (GLfloat * 16)(*identityMatrix)
m3dRotationMatrix44(RY, currentTime * m3dDegToRad(13.0), 0.0, 1.0, 0.0)
mv_matrix = m3dMultiply(T, m3dMultiply(RY, RX))
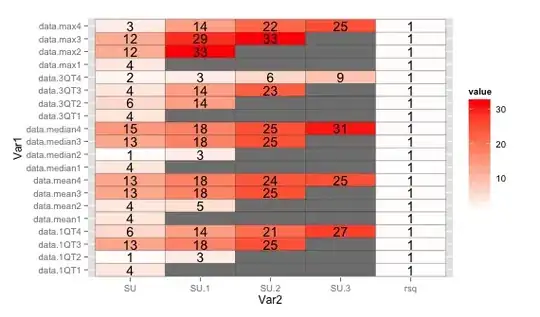
Note, if you want to use numpy.matmul
instead of m3dMultiply
, then you've to .reshape
the 16 element array to a 2 dimensional 4x4 array. e.g.:
R = np.matmul(np.array(RX).reshape(4,4), np.array(RY).reshape(4,4))
mv_matrix = np.matmul(R, np.array(T).reshape(4,4))
Or numpy.matrix
and the *
-operator:
mv_matrix = np.matrix(RX).reshape(4,4) * np.matrix(RY).reshape(4,4) * np.matrix(T).reshape(4,4)
See also Python: How to get cube to spin and move in OpenGL Superbible example