I got a decent result using the Hough transform for circles. This is the pipeline:
img = cv2.imread('I7Ykpbs.jpg', 0)
img = cv2.GaussianBlur(img, (5, 5), 2, 2)
img_th = cv2.adaptiveThreshold(img, 255, cv2.ADAPTIVE_THRESH_GAUSSIAN_C,
cv2.THRESH_BINARY, 9, 3)
circles = cv2.HoughCircles(img_th, cv2.HOUGH_GRADIENT, 2, minDist=30,
param1=200, param2=40, minRadius=10, maxRadius=20)
for i in range(circles.shape[1]):
c = circles[0,i,:]
center = (np.round(c[0]), np.round(c[1]))
radius = np.round(c[2])
# print(center)
# print(radius)
if np.linalg.norm(np.array([600., 600.])-center) < 500.:
cv2.circle(img, center, 3, (0,255,0), -1, 8, 0)
cv2.circle(img, center, radius, (0,0,255), 3, 8, 0)
plt.imshow(img)
plt.show()
It's not perfect but I think you can start from here and do some finetuning on parameters and preprocessing to optimize the result.
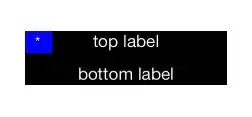