I think you want something like this to avoid any dependency on PIL/Pillow:
#!/usr/bin/env python3
import numpy as np
from PIL import Image
def colorize(im,black,white):
"""Do equivalent of PIL's "colorize()" function"""
# Pick up low and high end of the ranges for R, G and B
Rlo, Glo, Blo = black
Rhi, Ghi, Bhi = white
# Make new, empty Red, Green and Blue channels which we'll fill & merge to RGB later
R = np.zeros(im.shape, dtype=np.float)
G = np.zeros(im.shape, dtype=np.float)
B = np.zeros(im.shape, dtype=np.float)
R = im/255 * (Rhi-Rlo) + Rlo
G = im/255 * (Ghi-Glo) + Glo
B = im/255 * (Bhi-Blo) + Blo
return (np.dstack((R,G,B))).astype(np.uint8)
# Create black-white left-right gradient image, 256 pixels wide and 100 pixels tall
grad = np.repeat(np.arange(256,dtype=np.uint8).reshape(1,-1), 100, axis=0)
Image.fromarray(grad).save('start.png')
# Colorize from green to magenta
result = colorize(grad, [0,255,0], [255,0,255])
# Save result - using PIL because I don't know skimage that well
Image.fromarray(result).save('result.png')
That will turn this:

into this:
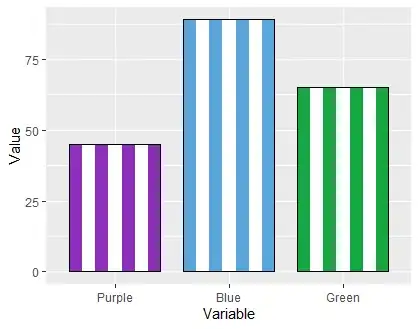
Note that this is the equivalent of ImageMagick's -level-colors BLACK,WHITE
operator which you can do in Terminal like this:
convert input.png -level-colors lime,magenta result.png
That converts this:
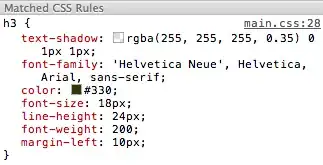
into this:
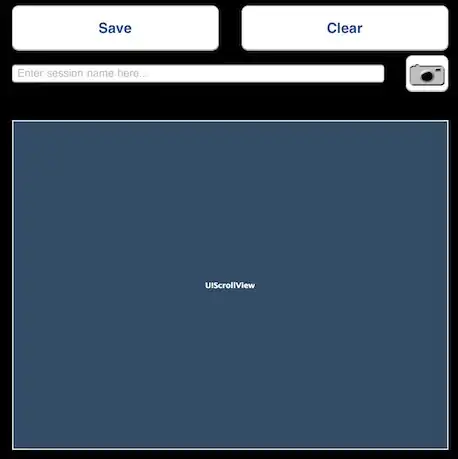
Keywords: Python, PIL, Pillow, image, image processing, colorize, colorise, colourise, colourize, level colors, skimage, scikit-image.