You can achieve the same width for the children just using Flow
like this:
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<View
android:id="@+id/view1"
android:layout_width="0dp"
android:layout_height="100dp"
android:background="#333" />
<View
android:id="@+id/view2"
android:layout_width="0dp"
android:layout_height="100dp"
android:background="#333" />
<View
android:id="@+id/view3"
android:layout_width="0dp"
android:layout_height="100dp"
android:background="#333" />
<View
android:id="@+id/view4"
android:layout_width="0dp"
android:layout_height="100dp"
android:background="#333" />
<androidx.constraintlayout.helper.widget.Flow
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="8dp"
app:constraint_referenced_ids="view1,view2,view3,view4"
app:flow_horizontalGap="8dp"
app:flow_maxElementsWrap="2"
app:flow_verticalGap="8dp"
app:flow_wrapMode="chain"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
Preview:
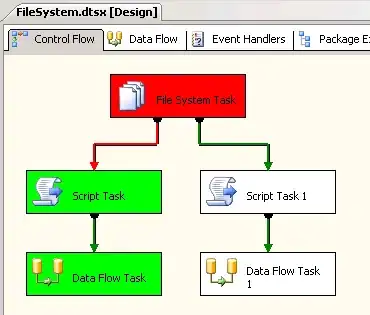
Note: One downside in this solution is, if you have an odd number of elements, then the last element will fill the full width. You can programatically set the last element width at runtime.