Using shiny you can do as follows:
library(shiny)
library(leaflet)
ui <- fluidPage(
leafletOutput('map'),
# Add custom CSS & Javascript;
tags$style(".leaflet-control-layers-expanded{color: red}")
)
server <- function(input, output, session) {
output$map <- renderLeaflet({
leaflet(quakes) %>%
addTiles() %>%
addMarkers() %>%
addLayersControl(
baseGroups = c("OSM (default)", "Toner", "Toner Lite"),
overlayGroups = c("Quakes", "Outline"),
options = layersControlOptions(collapsed = FALSE)
)
})
}
shinyApp(ui, server)
The key line being tags$style(".leaflet-control-layers-expanded{color: red}")
. Edit red as required using a Colour Name, Hex Colour Code, or RGB Colour Code.
Update
To display within the RStudio Viewer pane (i.e. without shiny) you can do the following:
library(leaflet)
library(htmltools)
m <- leaflet(quakes) %>%
addTiles() %>%
addMarkers() %>%
addLayersControl(
baseGroups = c("OSM (default)", "Toner", "Toner Lite"),
overlayGroups = c("Quakes", "Outline"),
options = layersControlOptions(collapsed = FALSE)
)
browsable(
tagList(
tags$style(".leaflet-control-layers-expanded{color: red}"),
m
)
)
Adapted from here.
Further Update
Based on the need for different colours:
As an aside form.leaflet-control-layers-list
is an alternative for .leaflet-control-layers-expanded
Within that there is a split between .leaflet-control-layers-base
and .leaflet-control-layers-overlays
as below:
library(shiny)
library(leaflet)
ui <- fluidPage(
leafletOutput('map'),
# Add custom CSS & Javascript;
tags$style(".leaflet-control-layers-base{color: red}",
".leaflet-control-layers-overlays{color: blue}")
)
server <- function(input, output, session) {
output$map <- renderLeaflet({
leaflet(quakes) %>%
addTiles() %>%
addMarkers() %>%
addLayersControl(
baseGroups = c("OSM (default)", "Toner", "Toner Lite"),
overlayGroups = c("Quakes", "Outline"),
options = layersControlOptions(collapsed = FALSE)
)
})
}
shinyApp(ui, server)
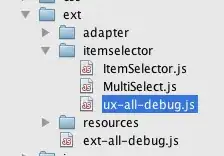
Unfortunately, I have not found how to colour by specific lines as the css does not seem to differentiate.