You should try setting the android:gravity
attribute correctly, and it has to work:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="fill_parent"
android:layout_height="fill_parent">
<LinearLayout android:gravity="bottom|center_horizontal"
android:layout_width="fill_parent" android:layout_height="wrap_content">
<ImageView android:id="@+id/calendarheader" android:src="@color/blue_start"
android:layout_width="wrap_content" android:layout_height="wrap_content"
android:minWidth="250dp" android:minHeight="30dp">
</ImageView>
</LinearLayout>
<GridView android:id="@+id/calendar" android:numColumns="7"
android:layout_gravity="top"
android:layout_width="fill_parent" android:layout_height="wrap_content"
android:background="@color/blue_start">
</GridView>
</LinearLayout>
i'm using here simple color background, and there is no gap:
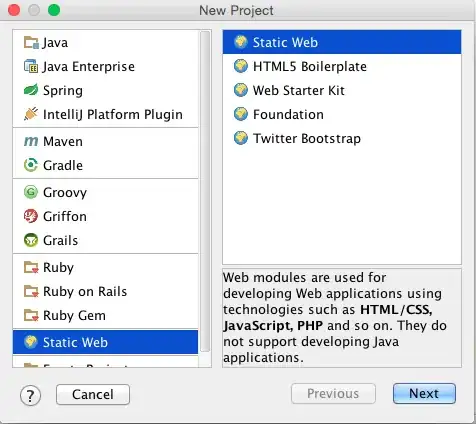
If you still having the problem, then you should see your blue_bg_with_text2
image, whether it has any empty pixels at the bottom.
ps: ignore the android:minWidth="250dp" android:minHeight="30dp"
part, it is there to imitate an image with that size.
Update
Here is the same view using RelativeLayout (it's a bit clearer at least for me):
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="fill_parent"
android:layout_height="fill_parent">
<Button android:id="@+id/selectedDayMonthYear"
android:textColor="#000000" android:layout_gravity="center"
android:textAppearance="?android:attr/textAppearanceMedium"
android:layout_width="0px" android:layout_height="0px" />
<ImageView android:id="@+id/prevMonth" android:src="@drawable/cal_left_arrow_off2"
android:layout_width="wrap_content" android:layout_height="wrap_content" />
<ImageView android:id="@+id/nextMonth" android:src="@drawable/cal_right_arrow_off2"
android:layout_width="wrap_content" android:layout_height="wrap_content"
android:layout_alignParentRight="true" />
<Button android:id="@+id/currentMonth" android:layout_weight="0.6"
android:textAppearance="?android:attr/textAppearanceMedium"
android:background="@drawable/calendar_bar2" android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_toRightOf="@id/prevMonth"
android:layout_toLeftOf="@id/nextMonth"
android:layout_alignBottom="@id/prevMonth"
android:layout_alignTop="@id/prevMonth" />
<ImageView android:id="@+id/calendarheader" android:src="@drawable/calendarheader"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:minHeight="25dp"
android:layout_centerInParent="true"
android:layout_below="@id/currentMonth"
android:layout_margin="0dp"
android:scaleType="fitXY" />
<GridView android:id="@+id/calendar" android:numColumns="7"
android:layout_gravity="top" android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:layout_below="@id/calendarheader"
android:layout_margin="0dp"
android:background="@color/white_fader_end">
</GridView>
</RelativeLayout>
I've added to it my resources as background (two of them are actually screenshots from yours).
What i observed is, you must
- either use a static image for your
calendar header background, or,
- if you use an xml drawable, you have
to drop the stroke element from it / set its
android:width
to 0
otherwise it will add an unnecessary gap around your view's drawable, that is as wide as the stroke width you've specified.
my output using static images as backgrounds looks this way:
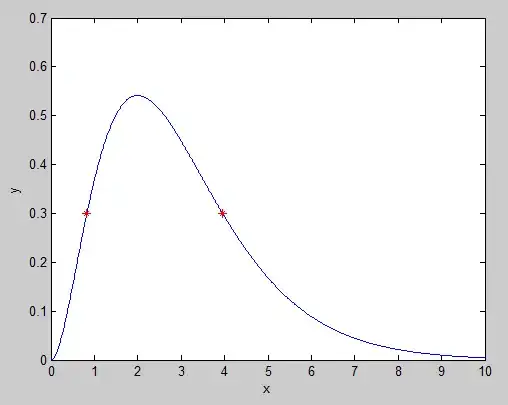
Update 2
The problem is not between your calendar header and gridView, but inside your gridView.
It has a 'padding' of 5 px inside (not real padding, see how it gets there):
The default selector
for the GridView
is a 9x9 patch rectangle with a border of 5px.
That 5 px is visible all around your gridView.
You can specify a different listSelector for this grid either by xml:
android:listSelector="@drawable/grid_selector"
or from code (onCreate):
gridView.setSelector(R.drawable.grid_selector);
Now if your grid_selector is an image or is a drawable xml without any margins, then the gap that causes the problem is gone.
You can also hide that gap, by setting a background color to your gridView, that matches or grandients the calendar header's background color.