You can use the following method to get the Digits in a different color
public SpannableString getColoredString(String string, int color){
SpannableString spannableString = new SpannableString(string);
for(int i = 0; i < string.length(); i++){
if(Character.isDigit(string.charAt(i))){
spannableString.setSpan(new ForegroundColorSpan(color), i, i+1, Spanned.SPAN_INCLUSIVE_INCLUSIVE);
}
}
return spannableString;
}
In your Activity code use the above function and set the return value to the textview
String text = String.format(Locale.getDefault(),"REMAINING TIME: %d MNS %d SECONDS ",(millisUntilFinished / 1000) / 60 ,(millisUntilFinished / 1000) % 60);
SpannableString string = getColoredString(text, Color.YELLOW);
remainingTime.setText(string);
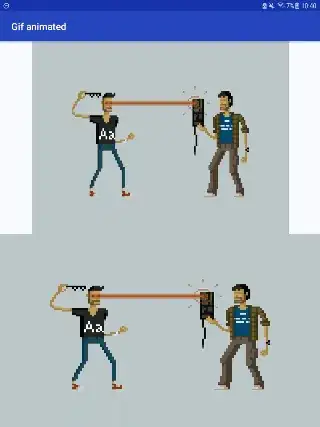