This simple expression or maybe a bit modified version of that might likely work on our input strings here:
[a-zA-Z]+\d+[a-zA-Z]+$|[a-zA-Z]+\d+
Test with re.findall
import re
regex = r"[a-zA-Z]+\d+[a-zA-Z]+$|[a-zA-Z]+\d+"
test_str = "a123123aas52342ooo345345ooo"
print(re.findall(regex, test_str))
Output
['a123123', 'aas52342', 'ooo345345ooo']
Test with re.finditer
import re
regex = r"[a-zA-Z]+\d+[a-zA-Z]+$|[a-zA-Z]+\d+"
test_str = "a123123aas52342ooo345345ooo"
matches = re.finditer(regex, test_str, re.MULTILINE)
for matchNum, match in enumerate(matches, start=1):
print ("Match {matchNum} was found at {start}-{end}: {match}".format(matchNum = matchNum, start = match.start(), end = match.end(), match = match.group()))
for groupNum in range(0, len(match.groups())):
groupNum = groupNum + 1
print ("Group {groupNum} found at {start}-{end}: {group}".format(groupNum = groupNum, start = match.start(groupNum), end = match.end(groupNum), group = match.group(groupNum)))
The expression is explained on the top right panel of this demo, if you wish to explore/simplify/modify it, and in this link, you can watch how it would match against some sample inputs step by step, if you like.
RegEx Circuit
jex.im visualizes regular expressions:
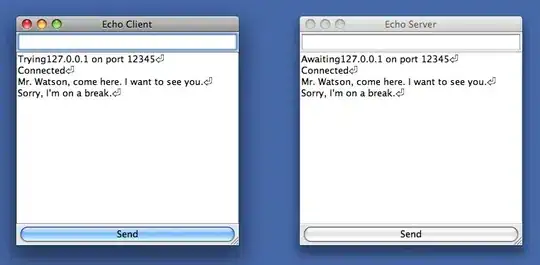