This is one way to do so:
- Traverse through the default tick-labels,
- Modify the required label to be bold faced,
- Reassign the tick-labels.
from matplotlib import rc
import pandas as pd
import matplotlib.pyplot as plt
rc('text', usetex=True)
df = pd.DataFrame(data={'value': [3, 5, 7, 4, 5]},
index=list('ABCDE'))
fig, ax = plt.subplots()
df.plot.barh(ax=ax)
fig.canvas.draw()
new_labels = []
to_modify = 'C'
for lab in ax.get_yticklabels():
txt = lab.get_text()
if txt == to_modify:
new_labels.append(r'$\textbf{%s}$' %txt) # append bold face text
else:
new_labels.append(txt) # append normal text
ax.set_yticklabels(new_labels)
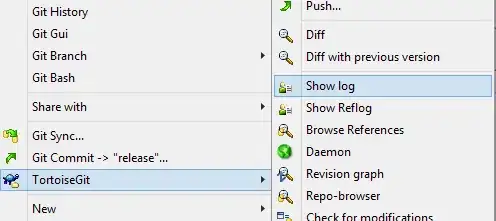
Alternative as suggested by ImportanceOfBeingEarnest : set_fontweight
only works if not using latex (TeX rendering).
fig, ax = plt.subplots()
df.plot.barh(ax=ax)
to_modify = 'C'
for lab in ax.get_yticklabels():
if lab.get_text() == to_modify:
lab.set_fontweight('bold')