I wrote this script using beautifulsoup4
and urllib
- so you'll need an internet connection for it to run.
import bs4 as bs
import urllib.request as request
repo = input('Please enter a repository name: ')
soup = bs.BeautifulSoup(request.urlopen(f'https://pypi.org/project/{repo}/'), 'lxml')
title = []
for item in soup.find_all('h1', {'class': 'package-header__name'}):
title.append(item.text)
version = title[0].split()[-1]
print(version)
This works because the title on the pypi website has the version in it, and has a html identifier that allows you to find the title easily. EG:
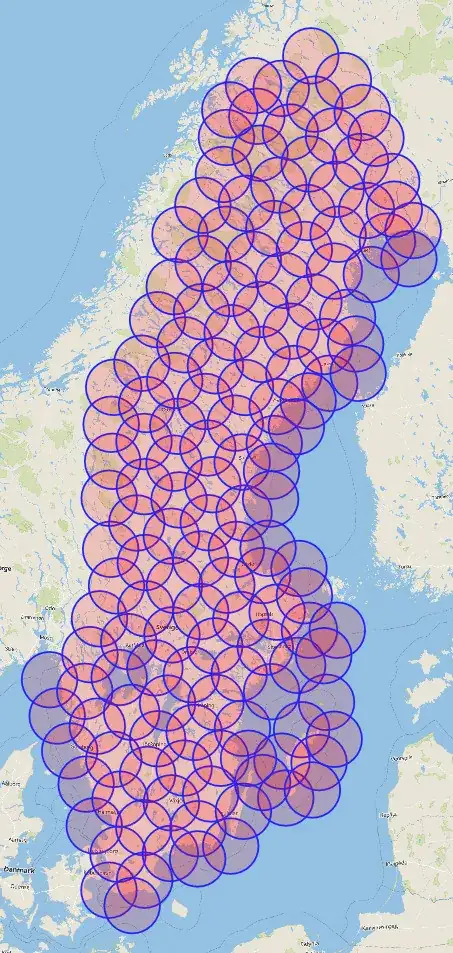
Sample run:
Please enter a repository name: pyaes
1.6.1
Also as a function:
def version(repo):
try:
soup = bs.BeautifulSoup(request.urlopen(f'https://pypi.org/project/{repo}/'), 'lxml')
title = []
for item in soup.find_all('h1', {'class': 'package-header__name'}):
title.append(item.text)
return title[0].split()[-1]
except urllib.error.URLError as e:
print('No internet connection')