I think you want a Perspective Transform with 4 points to go from this:
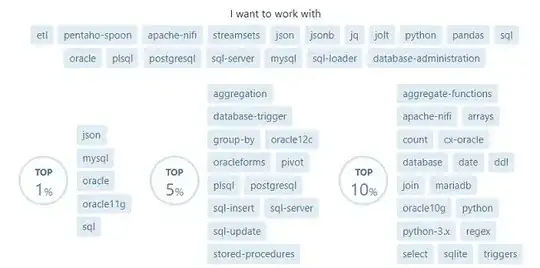
to this:
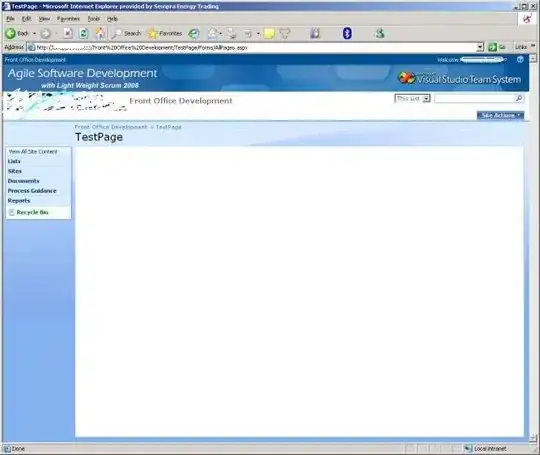
#!/usr/bin/env python3
import cv2
# Set width and height of output image
W, H = 600, 200
# Load input image
img = cv2.imread('speedo.png')
# Define points in input image: top-left, top-right, bottom-right, bottom-left
pts0 = np.float32([[337,300],[574,348],[567,378],[329,337]])
# Define corresponding points in output image
pts1 = np.float32([[0,0],[W,0],[W,H],[0,H]])
# Get perspective transform and apply it
M = cv2.getPerspectiveTransform(pts0,pts1)
result = cv2.warpPerspective(img,M,(W,H))
# Save reult
cv2.imwrite('result.png', result)
You can do the same thing much more simply with ImageMagick which is installed on most Linux distros and is available for macOS and Windows. In the Terminal, or Command Prompt, you just run:
magick speedo.png -distort perspective '337,300 0,0 574,348 200,0 567,378 200,100 329,337 0,100' -crop 200x100+0+0 result.png
Keywords: Python, command-line, shell, ImageMagick, OpenCV, distort, perspective, warp, affine, 4-point, 4-pt, transform, image, image processing.