It's not difficult to setup a ListView as the Control you showed.
You just need to paint some parts of its Items yourself.
Set:
1. ListView.OwnerDraw
= true
2. ListView.View
= View.Details
3. Add one Column, the size of the ListView minus the size of the ScrollBar (SystemInformation.VerticalScrollBarWidth
)
4. ListView.HeaderStyle
= none
if you don't want to show the header.
5. Subscribe to the ListView.DrawSubItem
event
6. Add an ImageList
, set its ImageSize.Height
to the height of the Items of your ListView and select it as the ListView.StateImageList
(so it won't be necessary to create a Custom Control to define the Items' height).
Here I've added an utility that selects the Text formatting style based on the current alignment of the Items' Text. It won't be necessary if you align the Text to the left only.
In case you have a very long list of Items to add to the ListView, a VirtualMode
is available.
It's not that different from the one you've shown, right?.
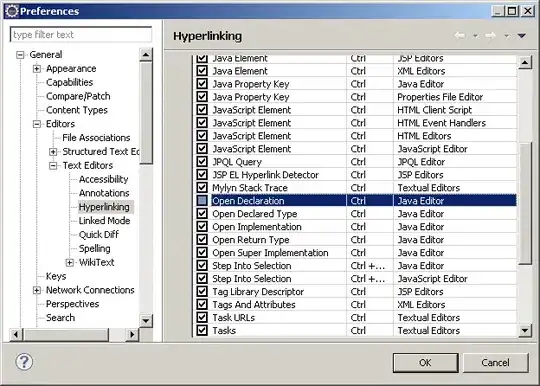
Color lvPanelsItemCurrentBackColor = Color.FromArgb(58, 188, 58);
Color lvPanelsItemSelectedBackColor = Color.FromArgb(48, 48, 48);
Color lvPanelsItemBackColor = Color.FromArgb(28,28,28);
Color lvPanelsItemForeColor = Color.White;
private void lvPanels_DrawSubItem(object sender, DrawListViewSubItemEventArgs e)
{
var lView = sender as ListView;
TextFormatFlags flags = GetTextAlignment(lView, e.ColumnIndex);
Color itemBackColor = lvPanelsItemBackColor;
Rectangle itemRect = e.Bounds;
itemRect.Inflate(-2, -2);
if (e.Item.Selected || e.Item.Focused) {
itemBackColor = e.Item.Focused ? lvPanelsItemCurrentBackColor : lvPanelsItemSelectedBackColor;
}
using (SolidBrush bkgrBrush = new SolidBrush(itemBackColor)) {
e.Graphics.FillRectangle(bkgrBrush, itemRect);
}
TextRenderer.DrawText(e.Graphics, e.SubItem.Text, e.SubItem.Font, e.Bounds, lvPanelsItemForeColor, flags);
}
private TextFormatFlags GetTextAlignment(ListView lstView, int colIndex)
{
TextFormatFlags flags = (lstView.View == View.Tile)
? (colIndex == 0) ? TextFormatFlags.Default : TextFormatFlags.Bottom
: TextFormatFlags.VerticalCenter;
flags |= TextFormatFlags.LeftAndRightPadding | TextFormatFlags.NoPrefix;
switch (lstView.Columns[colIndex].TextAlign)
{
case HorizontalAlignment.Left:
flags |= TextFormatFlags.Left;
break;
case HorizontalAlignment.Right:
flags |= TextFormatFlags.Right;
break;
case HorizontalAlignment.Center:
flags |= TextFormatFlags.HorizontalCenter;
break;
}
return flags;
}