tl;dr
LocalDate
.parse(
"15-August-2001" ,
DateTimeFormatter.ofPattern( "dd-MMMM-uuuu" , Locale.US )
)
.toString()
Tip: Better to exchange date-time data textually using only the standard ISO 8601 formats.
java.time
The Answer by Deadpool, while technically correct, is outdated. The modern approach uses the java.time classes that supplanted the legacy date-time classes with the adoption of JSR 310.
LocalDate
The LocalDate
class represents a date-only value without time-of-day and without time zone or offset-from-UTC.
DateTimeFormatter
Define a custom formatting pattern with DateTimeFormatter.ofPattern
. The formatting codes are not entirely the same as with the legacy SimpleDateFormat
class, so be sure to study the Javadoc carefully.
Specify a Locale
to determine the human language to use in translating the name of the month.
String input = "15-August-2001" ;
DateTimeFormatter f = DateTimeFormatter.ofPattern( "dd-MMMM-uuuu" , Locale.US ) ;
LocalDate ld = LocalDate.parse( input , f ) ;
System.out.println( "ld.toString(): " + ld ) ;
See this code run live at IdeOne.com.
ld.toString(): 2001-08-15
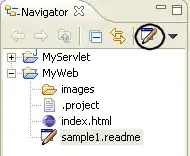