There can be 2 cases around it,
In case you simply want to add String
titles to each section
, implement UITableViewDataSource's
tableView(_: titleForHeaderInSection:)
method.
And if you want to give a custom view
for each section
, implement UITableViewDelegate's
tableView(_:viewForHeaderInSection:)
method.
Here is an example with tableView(_: titleForHeaderInSection:)
,
class VC: UIViewController, UITableViewDataSource {
let data = [["1","2","3"], ["4","5"]]
let sectionNames = ["This is Sec-1", "And this is Sec-2"]
func numberOfSections(in tableView: UITableView) -> Int {
return data.count
}
func tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return data[section].count
}
func tableView(_ tableView: UITableView, cellForRowAt indexPath: IndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCell(withIdentifier: "cell", for: indexPath) as! CustomCell
cell.textLabel?.text = data[indexPath.section][indexPath.row]
return cell
}
func tableView(_ tableView: UITableView, titleForHeaderInSection section: Int) -> String? {
return sectionNames[section]
}
}
Do implement tableView(_:heightForHeaderInSection:)
in case you need custom height for each section
.
Screenshot:
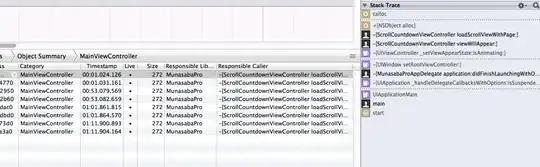
Edit:
Use accessoryType
as .checkmark
for cell selection.
Create a custom UITableViewCell
and override setSelected(_:animated:)
method in that like so,
class CustomCell: UITableViewCell {
override func setSelected(_ selected: Bool, animated: Bool) {
super.setSelected(selected, animated: animated)
self.accessoryType = selected ? .checkmark : .none
}
}
Set the reuseIdentifier
of CustomCell
in xib
as cell
. Also, update the tableView(_:cellForRowAt:)
method to dequeue CustomCell
instance. I updated that in the code above.