If you are interested in the last number, don't store the intermediate results.
I just tried it (using sys.getsizeof()
) and the sum of all integer values in the list would be 46308778320 bytes. Which is 46 GByte.
Even though the 1000000th Fibobacci number only has 92592 bytes
There is no limit to how big an integer can be in Python.
This is how the size of the integers grows:
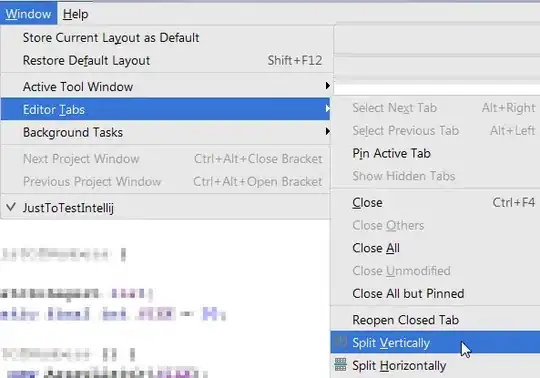
A small integer in Python has already has 28 bytes, which is quite large compared to a C int.
More info on this can be found at "sys.getsizeof(int)" returns an unreasonably large value?
How to calculate the total size?
import sys
fib = None
size_fib = 0
for _ in range(1000000):
fib = ... # calculation here
size_fib += sys.getsizeof(fib)
print(size_fib)