I suggest going for a ListView
in Details View
instead.
This is a more modern control, much more powerful and also more supportive when it comes to adding some extra styling..
ListView
has a BackgroundImage
which alone may be good enough. It doesn't support transparency, though.
But with a few tricks you can make it fake it by copying the background that would shine through..:
void setLVBack(ListView lv)
{
int alpha = 64;
Point p1 = lv.Parent.PointToScreen(lv.Location);
Point p2 = lv.PointToScreen(Point.Empty);
p2.Offset(-p1.X, -p1.Y );
if (lv.BackgroundImage != null) lv.BackgroundImage.Dispose();
lv.Hide();
Bitmap bmp = new Bitmap(lv.Parent.Width, lv.Parent.Height);
lv.Parent.DrawToBitmap(bmp, lv.Parent.ClientRectangle);
Rectangle r = lv.Bounds;
r.Offset(p2.X, p2.Y);
bmp = bmp.Clone(r, PixelFormat.Format32bppArgb);
using (Graphics g = Graphics.FromImage(bmp))
using (SolidBrush br = new SolidBrush(Color.FromArgb(alpha, lv.BackColor)))
{
g.FillRectangle(br, lv.ClientRectangle);
}
lv.BackgroundImage = bmp;
lv.Show();
}
A few notes:
- I hide the listview for a short moment while getting the background pixels
- I calculate an offset to allow borders; one could (and maybe should?) also use
SystemInformation.Border3DSize.Height
etc..
- I crop the right area using a
bitmap.Clone
overload
- finally I paint over the image with the background color of the LV, green in my case
- you can set the alpha to control how much I paint over the image
- Also note that I dispose of any previous image, so you can repeat the call when necessary, e.g. when sizes or positions change or the background etc..
- The
ListView
overlaps a PictureBox
(left) but sits on a TabPage
with an image of its own.
Result:
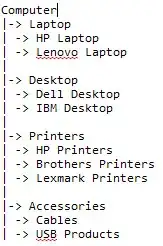