I would like to add to the existing answer that you should not compare secrets (such as passwords) using the equality operator due to timing attacks. PHP has a function specifically designed to avoid this: hash_equals()
if ($auth == 1) {
if (!hash_equals(md5($_SERVER['PHP_AUTH_USER']), $user1) || !hash_equals(md5($_SERVER['PHP_AUTH_PW']), $pass1)) {
header("WWW-Authenticate: Basic");
header("HTTP/1.0 401 Unauthorized");
die("Unauthorized access!");
}
}
I would also advise against MD5 as it's quite a weak algorithm that is not sufficient for passwords. Using a free online tool it is ridiculusly easy to "reverse" those hashes using lookup (rainbow) tables that contain a large number of hashes and their plain text equivalents, which can be queried within seconds.
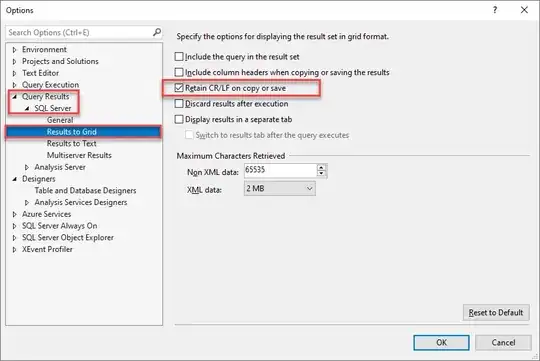
Should you chose to improve the security of your script I would suggest taking a look at password_hash
and it's pair password_verify()
which is timing attack safe.
You could make a standalone script that generates the hashes for you:
<form method="POST">
<input name="data[Username]" placeholder="Username">
<input name="data[Password]" placeholder="Password">
<input type="submit">
</form>
<?php
if (!empty($_POST['data']) && is_array($_POST['data'])){
$hashes = array_map(function ($value) {
return password_hash($value, PASSWORD_DEFAULT);
}, $_POST['data']);
echo '<p>Your hashes:</p><pre>';
print_r($hashes);
echo '</pre>';
}
This will give you two inputs that you can enter your desired values into and it will output hashes when you submit the form. These hashes are non-reversible, similar to MD5, but they are much less prone to the lookup attack demonstrated above.
![Input username Input password Submit button Your hashes: Array ( [Username] => $2y$10$5dt0CX73JcgLfAbrMAuFdeboEl5SQzkFDf9Uqwr/5I7OQaJdNiUl2 [Password] => $2y$10$E/fhcug/XuA0bQ5SVJXFS.b9nxeLpPM5r.hgCHcyVXx/4P6/u4QSO )](../../images/3863940300.webp)
You may notice that even when hitting refresh in your browser the values won't be the same each time. This is due to salting which further increases the resilience of any hashing algorithm by making sure you can't just take the hash and compare it against a table of known hashes with their plain text equivalents. Use the values you get in place of the MD5 hashes of your script, and replace the verification code with the following:
if ($auth == 1) {
if (!password_verify($_SERVER['PHP_AUTH_USER'], $user1) || !password_verify($_SERVER['PHP_AUTH_PW'], $pass1)) {
header("WWW-Authenticate: Basic");
header("HTTP/1.0 401 Unauthorized");
die("Unauthorized access!");
}
}