Using JSpinner
s for time input is simple enough and makes the implementation more robust.
The following example is a one-file MRE (copy and paste the entire code into SwingTest.java
and run):
import java.awt.BorderLayout;
import java.awt.GridLayout;
import java.time.LocalTime;
import java.time.format.DateTimeFormatter;
import java.time.temporal.ChronoUnit;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JSpinner;
import javax.swing.SpinnerNumberModel;
import javax.swing.Timer;
public class SwingTest extends JFrame {
public SwingTest() {
getContentPane().add(new MainPanel());
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLocationRelativeTo(null);
pack();
setVisible(true);
}
public static void main(String[] args) {
new SwingTest();
}
}
class MainPanel extends JPanel{
private final Timer timer;
MainPanel() {
setBorder(BorderFactory.createEmptyBorder(5,5,5,5));
setLayout(new BorderLayout(10,10));
TimePanel tp = new TimePanel();
add(tp);
JButton btn = new JButton("Open a window at selected time");
btn.addActionListener(e -> processTime(tp.getTime()));
add(btn, BorderLayout.PAGE_END);
timer = new Timer(0, e->openNewWindow());
timer.setRepeats(false);
}
private void processTime(LocalTime time) {
if(time == null || timer.isRunning()) return;
LocalTime now = LocalTime.now();
long delayInSeconds = ChronoUnit.SECONDS.between(now, time);
if (delayInSeconds < 0) { //if time is before now
delayInSeconds += 1440; //add 24X60 seconds
}
System.out.println("opening a window in "+ delayInSeconds + " seconds");
timer.setInitialDelay((int) (delayInSeconds * 1000));
timer.start();
}
private void openNewWindow() {
timer.stop();
JOptionPane.showMessageDialog(this, "New Window Opened !");
}
}
class TimePanel extends JPanel {
JSpinner hours, minutes, secconds;
TimePanel() {
hours = new JSpinner(new SpinnerNumberModel(12, 0, 24, 01));
minutes = new JSpinner(new SpinnerNumberModel(0, 0, 60, 01));
secconds = new JSpinner(new SpinnerNumberModel(0, 0, 60, 01));
setLayout(new GridLayout(1, 3, 10, 10));
add(hours);
add(minutes);
add(secconds);
}
LocalTime getTime(){
String h = String.valueOf(hours.getValue());
String m = String.valueOf(minutes.getValue());
String s = String.valueOf(secconds.getValue());
StringBuilder time = new StringBuilder();
time.append(h.length() < 2 ? 0+h: h).append(":")
.append(m.length() < 2 ? 0+m: m).append(":")
.append(s.length() < 2 ? 0+s: s);
System.out.println(time.toString());
return LocalTime.parse(time.toString(), DateTimeFormatter.ofPattern("HH:mm:ss"));
}
}
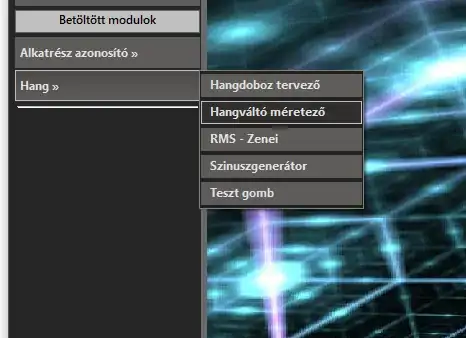