Normally you would use Negative Lookbehind for such situations, but since it can not be used in your situation, you can perhaps go around it like this:
([^ \\])\$0([^ \\])
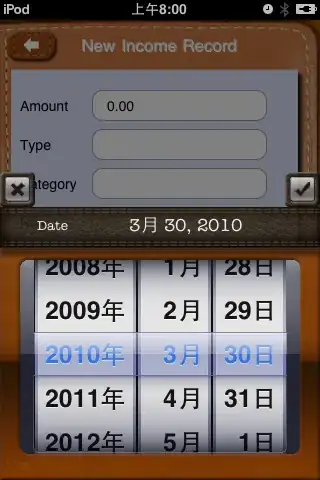
Regex demo - top right you can see description of individual regex components.
The thinking behind this is to separate '$0'
into three parts - ' + $0 + '
. The opening and closing braces are in their own groups - this allows you to extract the symbol that precedes and succeeds the $0 that you want to replace (you will see why this matters soon). We do not match cases where there is a \
or whitespace preceding the $0
. This allows us to capture the entire $0
together with the surrounding symbols, whatever they might be (e.g. '', "", [], ()
).
Inside your code, you replace the $0
with the word World
, surrounded by the symbols surrounding the original $0
- this is possible, since we originally captured them as groups. Your code therefore will be:
let string = "Hello '$0'. '$0' becomes the World. This shall remain \$0";
string = string.replace(/([^ \\])\$0([^ \\])/g, "$1World$2");
Bonus: some example results:
'' -> Hello 'World'. 'World' becomes the World. This shall remain $0
"" -> Hello "World". "World" becomes the World. This shall remain $0
[] -> Hello [World]. [World] becomes the World. This shall remain $0