Output: (All have exact same height)
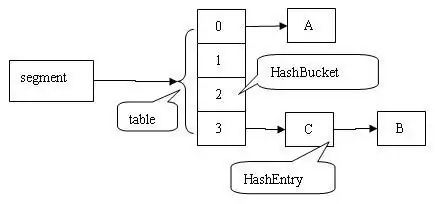
I think the best way to do it is to first find out height of TextField
, and then use it for your RaisedButton
, here is the full example code demonstrating the same.
void main() => runApp(MaterialApp(home: HomePage()));
class HomePage extends StatefulWidget {
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> with WidgetsBindingObserver {
double _height = 56; // dummy height
GlobalKey _globalKey = GlobalKey();
@override
void initState() {
super.initState();
SchedulerBinding.instance.addPostFrameCallback((_) {
setState(() {
// height of the TextFormField is calculated here, and we call setState to assign this value to Button
_height = _globalKey.currentContext.size.height;
});
});
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(),
body: Padding(
padding: const EdgeInsets.all(20.0),
child: Column(
children: <Widget>[
TextField(
key: _globalKey,
decoration: InputDecoration(hintText: "Email Adress"),
),
TextField(decoration: InputDecoration(hintText: "Password")),
SizedBox(height: 12),
SizedBox(
width: double.maxFinite,
height: _height, // this is the height of TextField
child: RaisedButton(
onPressed: () {},
child: Text("LOGIN TO MY ACCOUNT"),
),
),
],
),
),
);
}
}