The activity's main window is always resized to make room for the soft
keyboard on screen.
But it has limitations when your application is in fullscreen mode. Android official documentation says:
If the window's layout parameter flags include FLAG_FULLSCREEN, this
value for softInputMode will be ignored; the window will not resize,
but will stay fullscreen.
Check for more info:doc
Retrieve the overall visible display size in which the window this
view is attached to has been positioned in. This takes into account
screen decorations above the window, for both cases where the window
itself is being position inside of them or the window is being placed
under then and covered insets are used for the window to position its
content inside. In effect, this tells you the available area where
content can be placed and remain visible to users.
So,We need to detect when keyboard open and hide and calculate its size as per view.Below is example code.
Example code:
Manifest:
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme"
tools:ignore="GoogleAppIndexingWarning">
<activity android:name=".MainActivity"
android:windowSoftInputMode="adjustResize">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
activity_main.xml:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:fitsSystemWindows="true"
android:id="@+id/frame"
android:layout_height="match_parent"
tools:context=".MainActivity">
<WebView
android:id="@+id/MyWebView1"
android:layout_width="match_parent"
android:layout_height="match_parent" />
</RelativeLayout>
MainActivity.java
public class MainActivity extends AppCompatActivity {
WebView webView;
RelativeLayout relativeLayout;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
requestWindowFeature(Window.FEATURE_NO_TITLE);
getWindow().addFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN);
getWindow().setSoftInputMode(WindowManager.LayoutParams.SOFT_INPUT_ADJUST_PAN);
setContentView(R.layout.activity_main);
relativeLayout=findViewById(R.id.frame);
webView=findViewById(R.id.MyWebView1);
this.getWindow().getDecorView().getViewTreeObserver().addOnGlobalLayoutListener(new ViewTreeObserver.OnGlobalLayoutListener() {
@Override
public void onGlobalLayout() {
Rect r = new Rect();
getWindow().getDecorView().getWindowVisibleDisplayFrame(r);
int height = relativeLayout.getContext().getResources().getDisplayMetrics().heightPixels;
int diff = height - r.bottom;
if (diff != 0) {
if (relativeLayout.getPaddingBottom() != diff) {
relativeLayout.setPadding(0, 0, 0, diff);
}
} else {
if (relativeLayout.getPaddingBottom() != 0) {
relativeLayout.setPadding(0, 0, 0, 0);
}
}
}
});
final String mimeType = "text/html";
final String encoding = "UTF-8";
String html = "<html>\n" +
" <body style=\"padding: 0; margin: 0; box-sizing: border-box; border: 5px solid red; width: 100vw; height: 100vh;\">\n" +
" test\n" +
" <div style=\"width: 50vw; height: 70vh; border: 1px solid green;\">some div</div>\n" +
" <input placeholder=\"Type here and KB will cover this\">\n" +
" </body>\n" +
"</html>";
webView.loadDataWithBaseURL("", html, mimeType, encoding, "");
}
}
styles.xml:
<resources xmlns:tools="http://schemas.android.com/tools">
<style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar">
<!-- Customize your theme here. -->
<item name="colorPrimary">@color/colorPrimary</item>
<item name="colorPrimaryDark">@color/colorPrimaryDark</item>
<item name="colorAccent">@color/colorAccent</item>
</style>
</resources>
Output screen:
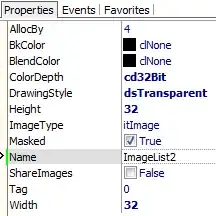