I made some tests and figured out that it's possible to use the browser's login autocomplete also for a user only field.
You may use this code for the form and set the position for the password field only if you need to show only the username field (read more at the end of the post, it's needed for Firefox):
<?php
$passwordFieldPositionAway = '';
$hidePasswordField = false; // change this accordingly with your needs, e.g. for Firefox set it to true
if($hidePasswordField)
{
$passwordFieldPositionAway = " style='position:absolute;top:-1000px;'";
}
?>
<html>
<head>
</head>
<body>
<form id="loginOnlyUsername" action="login.php" method="post">
<input type="text" name="username" autocomplete="username" placeholder="Username">
<input name="userPassword" type="password" <?= $passwordFieldPositionAway; ?> placeholder="password" autocomplete="current-password" value="anyString">
<input type="submit" value="Sign In!">
</form>
</body>
</html>
Tests I done
First, I modified the form suggested here.
index.php:
<html>
<head>
</head>
<body>
<form id="login" action="login.php" method="post">
<input type="text" name='username' autocomplete="username" placeholder='Username'>
<input name='userPassword' type="password" placeholder='password' autocomplete="current-password">
<input type="submit" value="Sign In!">
</form>
<form id="loginOnlyUsername" action="login.php" method="post">
<input type="text" name='username' autocomplete="username" placeholder='Username'>
<input name='userPassword' style='visibility: hidden; display:none;' type="password" placeholder='password' autocomplete="current-password">
<input type="submit" value="Sign In!">
</form>
</body>
</html>
login.php:
<?php
echo "<pre>";
print_r($_POST);
echo "</pre>";
?>
Chronium
Here are the steps I done with the Chronium browser:
At the first time I open the form, I insert my credentials:
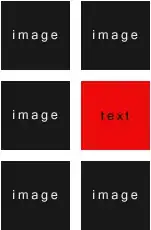
The browser prompt the dialog to save the credentials:
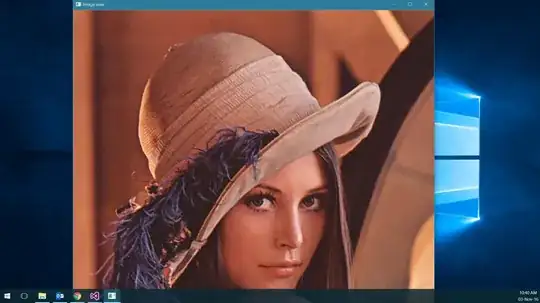
The PHP page receives these values:
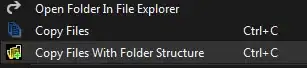
When I reopen the login page, the browser autofill both fields:

I make a login with different credentials:
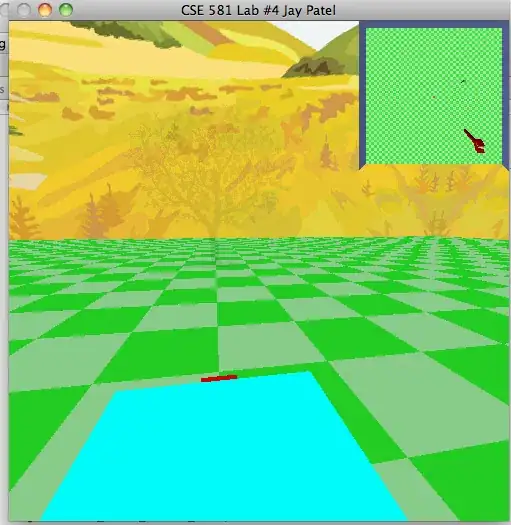
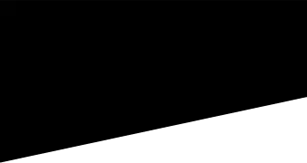
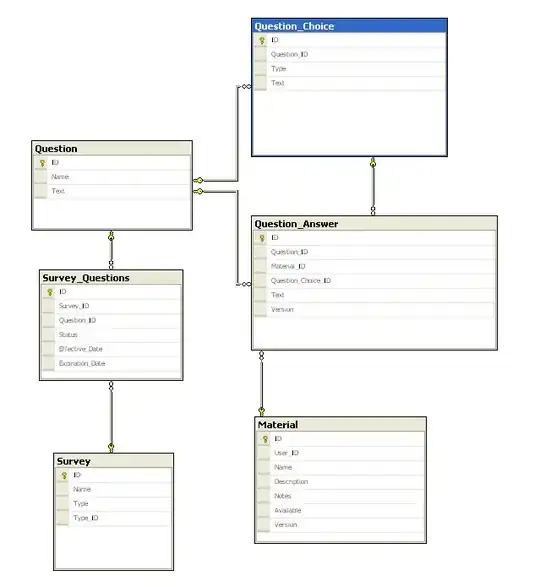
When reopen the login page, Chronium auto-fills both fields with the latest login data:

About the second form, which have the password field hidden, the browser's behavior is the same as the first form:

and it prompt to save the login also for that form.
Firefox
Firefox behaves differently:
Therefore, to make the user-only login works on Firefox, you may show the password field and prefill it with any string you want. To hide it in Firefox you must position it outside of the user's visible area in the page:
<form id="loginOnlyUsername" action="login.php" method="post">
<input type="text" name='username' autocomplete="username" placeholder='Username'>
<input name='userPassword' type="password" style='position:absolute;top:-1000px;' placeholder='password' autocomplete="current-password" value="anyString">
<input type="submit" value="Sign In!">
</form>
Some info:
- Chronium version:
77.0.3865.90 (Official Build) snap (a 64 bit)
- Firefox version:
68.0.2 (64-bit)
- Operative system: Ubuntu
18.04