The x-axis has appeared, but it seems that you're facing the same problem as described here. Anyway, I'll show you how you can get what you want and also avoid possible problems with the x-axis notations.
Plot 1:
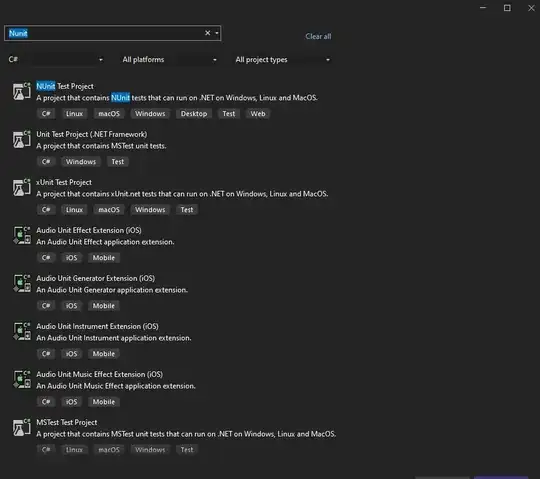
Code 1:
# imports
import matplotlib.ticker as ticker
import matplotlib.pyplot as plt
import pandas as pd
import numpy as np
# random data or other data sources
np.random.seed(123)
rows = 1440
df = pd.DataFrame(np.random.uniform(-1,1,size=(rows, 2)),
index=pd.date_range('1/1/2000', periods=1440),
columns=list('AB'))
df['A'] = df['A'].cumsum()
df['B'] = df['B'].cumsum()
# Plot
fig, ax = plt.subplots()
t = df.index
ax.plot(t, df['A'])
ax.plot(t, df['B'], color='red')
You can also edit and adjust the axis notations like this:
Plot 2:

Code 2:
# imports
import matplotlib.pyplot as plt
import pandas as pd
import numpy as np
# random data or other data sources
np.random.seed(123)
rows = 1440
df = pd.DataFrame(np.random.uniform(-1,1,size=(rows, 2)),
index=pd.date_range('1/1/2020', periods=1440),
columns=list('AB'))
df['A'] = df['A'].cumsum()
df['B'] = df['B'].cumsum()
# Make a list of empty myLabels
myLabels = ['']*len(df.index)
# Plot
fig, ax = plt.subplots()
t = df.index
ax.plot(t, df['A'])
ax.plot(t, df['B'], color='red')
# Set labels on every Nth element in myLabels specified by the interval variable
myLabels = ['']*len(df.index)
interval = 2
myLabels[::interval] = [item.strftime('%Y - %m - %d') for item in df.index[::interval]]
ax.xaxis.set_major_formatter(ticker.FixedFormatter(myLabels))
plt.gcf().autofmt_xdate()
# Tilt the labels
plt.setp(ax.get_xticklabels(), rotation=30, fontsize=10)
plt.show()