Date-time objects have no “format”
How to identify date format?
Date-time objects do not have a “format”. Each class defines its own mechanism for internally storing the date-time value. That mechanism is generally none of our business. The API of the class, the promises made by that class, are all that matters.
You may be conflating the text generated by a date-time object with the date-time object itself. Date-time classes can parse text as part of their instantiation. And date-time classes can generate text to represent their value. But the text and the object are separate and distinct.
Avoid Calendar
If I print out a calendar object I get:
The Calendar::toString
method is just a data-dump of many of its internal fields. Meant only for debugging obviously, not useful otherwise.
The Calendar
class and its commonly-used subclass GregorianCalendar
are both now legacy. They were supplanted years ago by the java.time classes as of the adoption of JSR 310. Never use them. GregorianCalendar
was specifically replaced by ZonedDateTime
.
Avoid Date
If I print out a Date object I get:
The Date::toString
method lies. That method dynamically applies the JVM’s current default time zone while generating text. A java.util.Date
actually represents a moment in UTC, not the time zone seen in the result of toString
. This anti-feature is one of many reasons to never use Date
.
The java.util.Date
class was replaced by java.time.Instant
, representing a moment in UTC as well but with a finer resolution of nanoseconds versus milliseconds.
ISO 8601
However I am examining the XML generated and I observe this format:
2014-02-28T08:00:00.000Z
The format of that text is defined in the ISO 8601 standard. This standard is wisely designed to represent a variety of date-time values textually for data-exchange. This standard supplants earlier poorly-defined date-time text formats, such as seen in the early email protocols.
The java.time classes use ISO 8601 formats by default when parsing/generating strings.
Current moment in UTC
To capture the current moment in UTC, use Instant
.
Instant instant = Instant.now() ; // Capture the current moment in UTC.
Generate text representing that value in standard ISO 8601 format. The Z
on the end means UTC, and is pronounced “Zulu”.
2020-01-23T12:34:56.123456Z
Parse such a string.
Instant instant = Instant.parse( "2020-01-23T12:34:56.123456Z" );
Time zone
See that same moment through the wall-clock time used by the people of a particular region, a time zone.
ZoneId z = ZoneId.of( "America/Montreal" ) ;
ZonedDateTime zdt = instant.atZone( z ) ;
Generate a string in a format that wisely extends the ISO 8601 standard by appending the name of the time zone in square brackets.
String output = zdt.toString() ;
See this code run live at IdeOne.com.
instant.toString(): 2020-01-23T12:34:56.123456Z
zdt.toString(): 2020-01-23T07:34:56.123456-05:00[America/Montreal]
Date-time types in Java
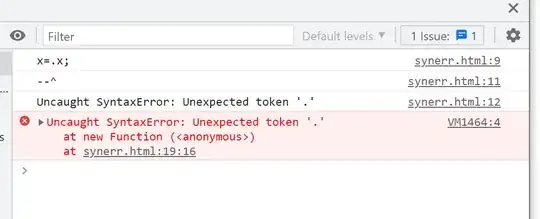
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.