Subclass your textfield and override the clearButtonRect
function
class CustomTextField: UITextField {
override func clearButtonRect(forBounds bounds: CGRect) -> CGRect {
return CGRect(x: xPos, y:yPos, width: yourWidth, height: yourHeight)
}
}
EDIT 1 - Subclass 3rd party Textfield
As you are using 3rd party library calls TextFieldEffects you will need to follow the below steps.
If you you are using storyboard and have a textfield on there (set the class to CustomTextField)
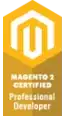
if you can doing it programmatically you will need to change it there.
Then create a new swift file call it CustomTextField.swift and add the below code
import UIKit
import TextFieldEffects // Import the 3rd party library
// Now you are subclassing the 3rd party textfield
// Change it to the textfield you are using and if you are using multiple create subclass for individual ones.
class CustomTextField: HoshiTextField {
override func clearButtonRect(forBounds bounds: CGRect) -> CGRect {
// change the return line as per your requirement.
return CGRect(x: 300, y: 25, width: 40, height: 20)
}
}
Output
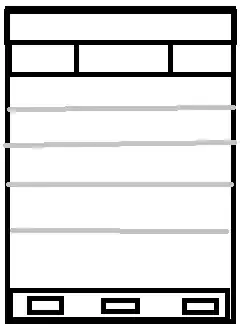
Hope this will help.