A subsequence of a sequence is called a slice and the operation that extracts a subsequence is called slicing. Like with indexing, we use square brackets ([ ]) as the slice operator, but instead of one integer value inside we have two, seperated by a colon (:):
>>> singers = "Peter, Paul, and Mary"
>>> singers[0:5]
'Peter'
>>> singers[7:11]
'Paul'
>>> singers[17:21]
'Mary'
>>> classmates = ("Alejandro", "Ed", "Kathryn", "Presila", "Sean", "Peter")
>>> classmates[2:4]
('Kathryn', 'Presila')
The operator [n:m] returns the part of the sequence from the n’th element to the m’th element, including the first but excluding the last. This behavior is counter-intuitive; it makes more sense if you imagine the indices pointing between the characters, as in the following diagram:
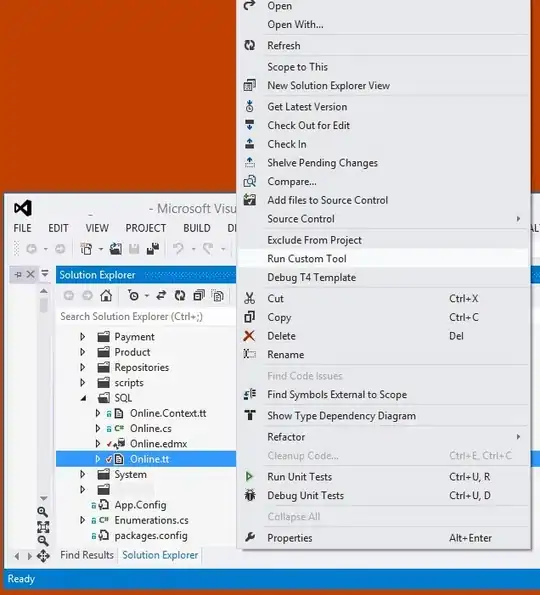
'banana' string
If you omit the first index (before the colon), the slice starts at the beginning of the string. If you omit the second index, the slice goes to the end of the string. Thus:
>>> fruit = "banana"
>>> fruit[:3]
'ban'
>>> fruit[3:]
'ana'
What do you think s[:] means? What about classmates[4:]?
Negative indexes are also allowed, so
>>> fruit[-2:]
'na'
>>> classmates[:-2]
('Alejandro', 'Ed', 'Kathryn', 'Presila')
source