You can directly use the element.files
property to access the files and use the Filreader class from dart:html
. I have created an example below to show you how a text file and image can be read. This example is based on FileReader examples in another post.
Accessing the file
Here element
is the FileUploadInputElement
reference.
element.files[0]
or in case of multiple files element.files
Set up your file reader
String option1Text = "Initialized text option1";
Uint8List uploadedImage;
FileUploadInputElement element = FileUploadInputElement()..id = "file_input";
// setup File Reader
FileReader fileReader = FileReader();
Use FileReader to read the file
fileReader.readAsText(element.files[0])
connect the listener for load event
fileReader.onLoad.listen((data) {
setState(() {
option1Text = fileReader.result;
});
});
connect error events
fileReader.onError.listen((fileEvent) {
setState(() {
option1Text = "Some Error occured while reading the file";
});
});
Use Image.memory
to show images from byte array.
Image.memory(uploadedImage)
Note: In the following example we choose a file and click the respective button to handle the file reading. But the same can be achieved by connecting the logic in respective events of the FileUploadInputElement
element in a similar approach. eg: element.onLoad.listen
or element.onError.listen
event streams.
Full Example
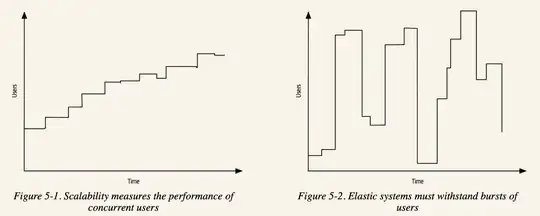
import 'dart:typed_data';
import 'package:flutter/material.dart';
import 'dart:ui' as ui;
import 'dart:html';
class FileUploadTester extends StatefulWidget {
@override
_FileUploadTesterState createState() => _FileUploadTesterState();
}
class _FileUploadTesterState extends State<FileUploadTester> {
String option1Text = "Initialized text option1";
Uint8List uploadedImage;
FileUploadInputElement element = FileUploadInputElement()..id = "file_input";
// setup File Reader
FileReader fileReader = FileReader();
// reader.onerror = (evt) => print("error ${reader.error.code}");
@override
Widget build(BuildContext context) {
ui.platformViewRegistry.registerViewFactory("add_input", (int viewId) {
return element;
});
return Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
crossAxisAlignment: CrossAxisAlignment.end,
children: <Widget>[
FlatButton(
color: Colors.indigoAccent,
child: Text('ReadFile'),
onPressed: () {
fileReader.onLoad.listen((data) {
setState(() {
option1Text = fileReader.result;
});
});
fileReader.onError.listen((fileEvent) {
setState(() {
option1Text = "Some Error occured while reading the file";
});
});
fileReader.readAsText(element.files[0]);
},
),
Expanded(
child: Container(
child: Text(option1Text),
),
),
Expanded(child: HtmlElementView(viewType: 'add_input')),
Expanded(
child: uploadedImage == null
? Container(
child: Text('Uploaded image should shwo here.'),
)
: Container(
child: Image.memory(uploadedImage),
),
),
FlatButton(
child: Text('Show Image'),
color: Colors.tealAccent,
onPressed: () {
fileReader.onLoad.listen((data) {
setState(() {
uploadedImage = fileReader.result;
});
});
fileReader.onError.listen((fileEvent) {
setState(() {
option1Text = "Some Error occured while reading the file";
});
});
fileReader.readAsArrayBuffer(element.files[0]);
},
),
],
);
}
}
Below