Life can be much simpler by using a CursorAdapter such as SimpleCursorAdapter when using ListViews.
They are designed to handle Cursors and there is no need for intermediate arrays and extracting it/them from cursors, they pass the id to the onItemClick Listeners (4th parameter) and that is all that is needed to pass a database row between activities.
The one inconvenience is that the id MUST be a column named _id (or renamed using AS e.g. SELECT *,id AS _id).
- this is defined as as Constant named _ID in BaseColumns (used in the example below).
Example
The following is a basic App, based upon your code (some deviations are commented)
DatabaseHandler.java
public class DatabaseHandler extends SQLiteOpenHelper {
public static final String DBNAME = "campsite.db";
public static final int DBVERSION = 1;
public static final String TABLE_CAMPSITES = "campsite";
public static final String COL_ID = BaseColumns._ID; //<<<<<<<<<< Important for Adapter
public static final String COL_NAME = "name";
public static final String COL_CITY = "city";
public static final String COL_FEATURE = "feature";
public static final String COL_FAVORITE = "favourite";
public static final String COL_RATING = "rating";
public static final String COL_LATITUDE = "latitude";
public static final String COL_LONGITUDE = "longitude";
public DatabaseHandler(@Nullable Context context) {
super(context, DBNAME, null, DBVERSION);
}
@Override
public void onCreate(SQLiteDatabase db) {
String CREATE_CAMPSITES_TABLE = "CREATE TABLE " + TABLE_CAMPSITES + "("
+ COL_ID + " INTEGER PRIMARY KEY," + COL_NAME + " TEXT,"
+ COL_CITY + " TEXT," + COL_FEATURE + " TEXT," + COL_FAVORITE + " TEXT," + COL_RATING + " INTEGER," + COL_LATITUDE + " REAL," + COL_LONGITUDE + " REAL" + ")";
db.execSQL(CREATE_CAMPSITES_TABLE);
}
public Cursor getCampsitesAsCursor() {
SQLiteDatabase db = this.getWritableDatabase();
return db.query(
TABLE_CAMPSITES, /* Table i.e. FROM part */
/* Columns */
new String[]{
COL_ID,
COL_NAME,
COL_CITY,
COL_FEATURE
},
null,null,null,null,null
);
}
public Cursor getCampSiteById(long id) {
SQLiteDatabase db = this.getWritableDatabase();
return db.query(TABLE_CAMPSITES,null,COL_ID + "=?",new String[]{String.valueOf(id)},null,null,null);
}
public long addCampsiteRow(String name, String city, String feature, String favourite, int rating, double latitude, double longitude) {
ContentValues cv = new ContentValues();
cv.put(COL_NAME,name);
cv.put(COL_CITY,city);
cv.put(COL_FEATURE, feature);
cv.put(COL_FAVORITE,favourite);
cv.put(COL_RATING,rating);
cv.put(COL_LATITUDE,latitude);
cv.put(COL_LONGITUDE,longitude);
SQLiteDatabase db = this.getWritableDatabase();
return db.insert(TABLE_CAMPSITES,name,cv);
}
@Override
public void onUpgrade(SQLiteDatabase db, int oldVersion, int newVersion) {
}
}
browse_row_layout.xml (Note your's could probably be used without any changes)
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="horizontal"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<TextView
android:id="@+id/campsiteName"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content">
</TextView>
<TextView
android:id="@+id/campsiteCity"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content">
</TextView>
<TextView
android:id="@+id/campsiteFeature"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content">
</TextView>
</LinearLayout>
Browse.java
public class Browse extends AppCompatActivity {
public static final String INTENTKEY_CAMPSITEID = "ik_campsiteID";
DatabaseHandler DBHandler;
ListView lvCampsites;
Cursor campsiteList;
SimpleCursorAdapter adapter;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_browse);
lvCampsites = this.findViewById(R.id.lvCampsites);
DBHandler = new DatabaseHandler(this);
addSometestingData();
manageListView();
}
private void manageListView() {
campsiteList = DBHandler.getCampsitesAsCursor();
if (adapter == null) {
adapter = new SimpleCursorAdapter(
this,
R.layout.browse_row_layout,campsiteList,
new String[]{
DatabaseHandler.COL_NAME,
DatabaseHandler.COL_CITY,
DatabaseHandler.COL_FEATURE},
new int[]{
R.id.campsiteName,
R.id.campsiteCity,
R.id.campsiteFeature},
0
);
lvCampsites.setAdapter(adapter);
lvCampsites.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intent = new Intent(view.getContext(),CampSiteInfo.class);
intent.putExtra(INTENTKEY_CAMPSITEID,id);
startActivity(intent);
}
});
} else {
adapter.swapCursor(campsiteList);
}
}
private void addSometestingData() {
if(DatabaseUtils.queryNumEntries(DBHandler.getWritableDatabase(),DatabaseHandler.TABLE_CAMPSITES) < 1) {
DBHandler.addCampsiteRow(
"Brownsea Island",
"Bournemouth",
"wet wet wet",
"might be",
10,1234567.567,890678.564
);
DBHandler.addCampsiteRow(
"Youbury",
"Oxford",
"Trees, tress and more trees",
"NO",
-100,66463636.8877, 42316867.3542
);
// etc
}
}
//<<<<<<<<<< ADDED >>>>>>>>>>
@Override
protected void onDestroy() {
campsiteList.close(); //<<<<<<<<<< closes the cursor when done with it
super.onDestroy();
}
//<<<<<<<<<< ADDED >>>>>>>>>>
@Override
protected void onResume() {
super.onResume();
manageListView(); //<<<<<<<<<< refreshes the listview if need be
}
}
Note that when instantiaing the adapter that the 4th parameter is a string array of the columns From which the data to be displayed is extracted and that the 5th parameter is an int array of the id's in the layout for each row that correspond to the 4th parameter.
As such the SimpleCursorAdapter is quite flexible (more so or more simpler than SimpleAdapter) in regards to what can be displayed.
CampSiteInfo.java
This has been included as it shows the basics of retrieve the data for the passed id, it logs just the name.
public class CampSiteInfo extends AppCompatActivity {
DatabaseHandler DBHandler;
Cursor csr;
long campSiteId;
int campSiteRating;
String campSiteName = "", campSiteCity = "", campSiteFeature = "", campSiteFavourite = "";
double campSiteLatitude = 0.0, campSiteLongitude = 0.0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_camp_site_info);
campSiteId = this.getIntent().getLongExtra(Browse.INTENTKEY_CAMPSITEID,-1);
DBHandler = new DatabaseHandler(this);
csr = DBHandler.getCampSiteById(campSiteId);
if (csr.moveToFirst()) {
campSiteName = csr.getString(csr.getColumnIndex(DatabaseHandler.COL_NAME));
campSiteCity = csr.getString(csr.getColumnIndex(DatabaseHandler.COL_CITY));
campSiteFeature = csr.getString(csr.getColumnIndex(DatabaseHandler.COL_FEATURE));
campSiteFavourite = csr.getString(csr.getColumnIndex(DatabaseHandler.COL_FAVORITE));
campSiteRating = csr.getInt(csr.getColumnIndex(DatabaseHandler.COL_RATING));
campSiteLatitude = csr.getDouble(csr.getColumnIndex(DatabaseHandler.COL_LATITUDE));
campSiteLongitude = csr.getDouble(csr.getColumnIndex(DatabaseHandler.COL_LONGITUDE));
}
Log.d("CAMPSITEINFO","CampsiteInfo activity started with campsite Name as " + campSiteName );
// .... code
}
@Override
protected void onDestroy() {
if (!csr.isClosed()) {
csr.close();
}
super.onDestroy();
}
}
Result
When run the 2 rows are displayed :-
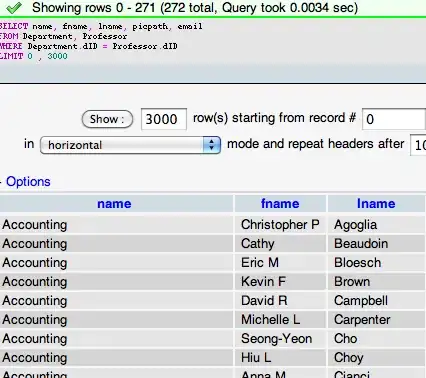
Clicking a row (Brownsea Island) then the CampSiteInfo is started (just a blank display):-
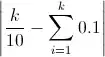
and the log contains :-
2019-09-27 12:53:42.332 24769-24769/aso.asocamper D/CAMPSITEINFO: CampsiteInfo activity started with campsite Name as Brownsea Island
i.e. The correct id has been passed and the Name, which wasn't passed via the intent has been retrieved.