That spacing is part of the Android default Drawable background for the Buttons.
You can remove this by changing the color, as this will replace the background. As you just did.
If you want to remove the space without changing the color explicitly you could set the theme in the Android project on the styles.xml file and use the Android color from the button, something like this:
<style name="NoPaddingButton" parent="android:Widget.Material.Button">
<item name="android:layout_margin">0dip</item>
<item name="android:background">@color/button_material_light</item>
</style>
Then add this style to the Theme, usually called MainTheme.Base
<item name="android:buttonStyle">@style/NoPaddingButton</item>
The margin is part of this answer.
The only issue is that the above will remove the replay effect (the effect you see when you tap on the button).
To get it back you can follow this:
In the Drawable folder add a new XML file called button_gray_ripple.xml and paste the following code:
<?xml version="1.0" encoding="utf-8"?>
<!-- in drawable folder-->
<ripple
xmlns:android="http://schemas.android.com/apk/res/android"
android:color="?android:colorControlHighlight">
<item android:id="@android:id/mask">
<shape android:shape="rectangle">
<solid android:color="?android:colorAccent" />
</shape>
</item>
<item>
<shape android:shape="rectangle">
<solid android:color="@color/button_material_light" />
</shape>
</item>
</ripple>
Your previously created style will then look like this:
<style name="MyButton" parent="android:Widget.Material.Button">
<item name="android:layout_margin">0dip</item>
<item name="android:background">@drawable/button_gray_ripple</item>
</style>
All the above should look like this:
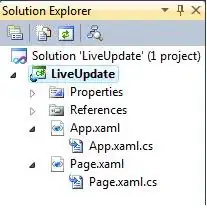