I'm not sure about the best way, yet I'm guessing that you wish to search/capture the checkstatus
s, for which we'd then start with a simple expression:
(?i)^https?://(?:w{3}\.)?example\.com/bar[12]/([^/]*)/?$
assuming that there would be optional wwww.
((?:w{3}\.)?
), http
or https
(s?
), and ending trailing slashes (/?
), which if not, we can simply remove those from the expression:
(?i)^http://example\.com/bar[12]/([^/]*)$
Test
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class RegularExpression{
public static void main(String[] args){
final String regex = "(?i)^https?://(?:w{3}\\.)?example\\.com/bar[12]/([^/]*)/?$";
final String string = "http://example.com/bar1/checkstatus\n"
+ "http://example.com/bar2/checkstatus\n"
+ "https://www.example.com/bar1/checkstatus\n"
+ "https://www.example.com/bar2/checkstatus\n"
+ "http://example.com/bar1/checkstatus/\n"
+ "http://example.com/bar2/checkstatus/\n"
+ "https://www.example.com/bar1/checkstatus/\n"
+ "https://www.example.com/bar2/checkstatus/";
final Pattern pattern = Pattern.compile(regex, Pattern.MULTILINE);
final Matcher matcher = pattern.matcher(string);
while (matcher.find()) {
System.out.println("Full match: " + matcher.group(0));
for (int i = 1; i <= matcher.groupCount(); i++) {
System.out.println("Group " + i + ": " + matcher.group(i));
}
}
}
}
Output
Full match: http://example.com/bar1/checkstatus
Group 1: checkstatus
Full match: http://example.com/bar2/checkstatus
Group 1: checkstatus
Full match: https://www.example.com/bar1/checkstatus
Group 1: checkstatus
Full match: https://www.example.com/bar2/checkstatus
Group 1: checkstatus
Full match: http://example.com/bar1/checkstatus/
Group 1: checkstatus
Full match: http://example.com/bar2/checkstatus/
Group 1: checkstatus
Full match: https://www.example.com/bar1/checkstatus/
Group 1: checkstatus
Full match: https://www.example.com/bar2/checkstatus/
Group 1: checkstatus
If you wish to simplify/modify/explore the expression, it's been explained on the top right panel of regex101.com. If you'd like, you can also watch in this link, how it would match against some sample inputs.
RegEx Circuit
jex.im visualizes regular expressions:
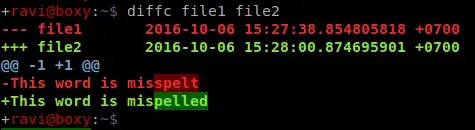