Combining with @SudhakarRS's answer:
var child = require('child_process').execFile('powershell', [
'(Get-Process SomeProcess).StartInfo.EnvironmentVariables'
], function(err, stdout, stderr) {
console.log(stdout);
});
If you want to debug it, make sure you peek at err
and stderr
.
Replacing SomeProcess
with notepad
works for me, but using notepad.exe
does not.
On powershell you can get the processes with a particular name using Get-Process [process name]
.
So, for example, if I have 4 instances of notepad running and do Get-Process notepad
, I see this:
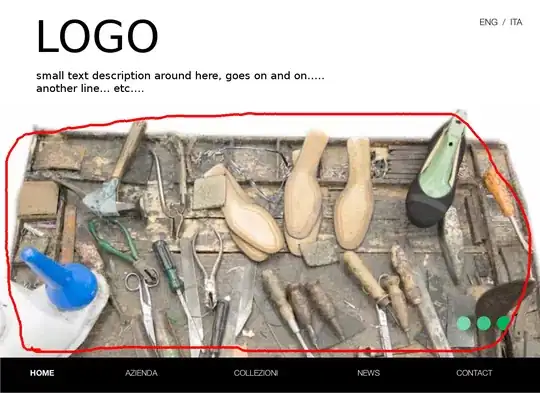
You can get the process IDs with (Get-Process notepad).Id
which returns:
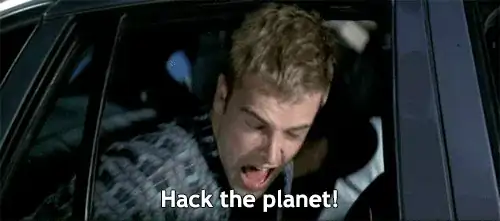
You could use the same code to choose the ID:
var child = require('child_process').execFile(
'powershell',
['(Get-Process notepad).Id'],
function(err, stdout, stderr) {
var ids = stdout.split("\r\n");
ids.pop(); //remove the blank string at the end
console.log(ids);
}
);
^ which returns:
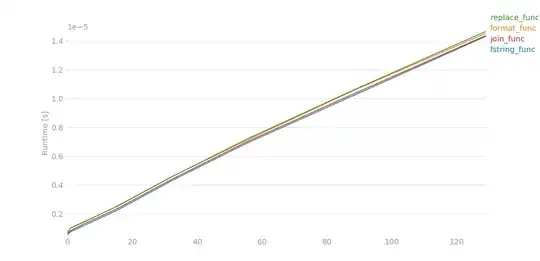
If you just want to grab the first process with a name, it's:
(Get-Process notepad)[0].StartInfo.EnvironmentVariables
^ obviously replace notepad
with your process name.