Thanks to @NicoH. this code is great. I used it to geoplot a TSP solver.
nyc_map_zoom = plt.imread('https://aiblog.nl/download/nyc_-74.3_-73.7_40.5_40.9.png')
def plot_on_map(df, BB, nyc_map, s=40, alpha=0.2):
x = df['Longitude']
y = df['Latitude']
# calculate position and direction vectors:
x0 = x.iloc[range(len(x)-1)].values
x1 = x.iloc[range(1,len(x))].values
y0 = y.iloc[range(len(y)-1)].values
y1 = y.iloc[range(1,len(y))].values
xpos = (x0+x1)/2
ypos = (y0+y1)/2
xdir = x1-x0
ydir = y1-y0
# plot map
fig, ax = plt.subplots(figsize=(20,20))
ax.scatter(x,y, marker='H',c='fuchsia',s=80,label=df["Name"])
ax.set_xlim((BB[0], BB[1]))
ax.set_ylim((BB[2], BB[3]))
ax.set_title('Pizza Locations and Route Directions', fontsize=15)
ax.imshow(nyc_map, zorder=0, extent=BB)
ax.plot(x,y,linewidth=3)
plt.legend(title='Pizza Joints', facecolor='white', framealpha=1,fontsize=15,title_fontsize=18, fancybox=True,edgecolor = 'k')
# plot arrow on each line:
for X,Y,dX,dY in zip(xpos, ypos, xdir, ydir):
ax.annotate("", xytext=(X,Y),xy=(X+0.001*dX,Y+0.001*dY),
arrowprops=dict(arrowstyle="->", linewidth=3,color='k'), size = 40)
plt.savefig('Pizza_Route.png',bbox_inches='tight');
BB_zoom = (-74.3, -73.7, 40.5, 40.9)
plot_on_map(TSP, BB_zoom, nyc_map_loc, s=20, alpha=0.3)
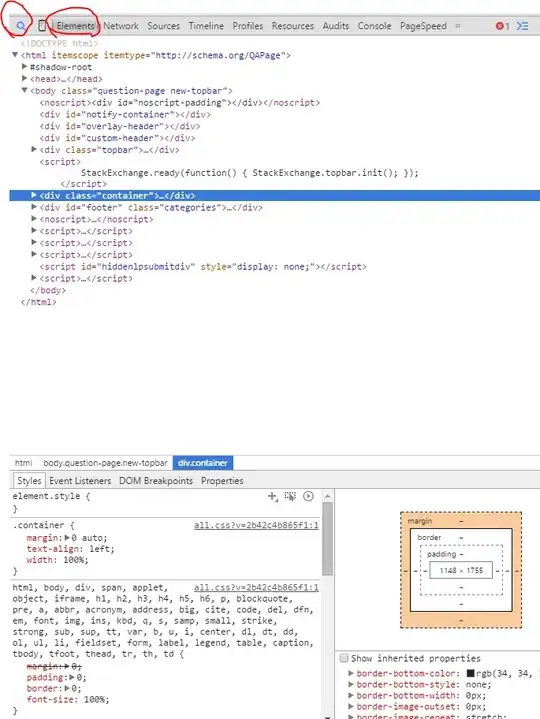