Each instance of a class or struct has a "personal memory space" for data, but methods are shared once for all objects.
First, you need 4 bytes on x32 or 8 bytes on x64 to store the reference to the memory address of the object (reference is a hidden pointer to forget to manage it).
Next, the object has two data members here:
- One integer that takes 4 bytes.
- One string that here takes 5 chars : 5x2 bytes = 10 bytes.
So for data the object takes 18 bytes on x32 or 22 bytes on x64 system.
Since string object contains an integer for the length, the size is a little more than that : 22 on x32 and 26 on x64.
Since string is a reference, we need to add again 4 or 8 bytes => 26 or 34 bytes.
Since string has some other static and instance fields in the class declaration like the first char, it takes a little more than this.
Is string actually an array of chars or does it just have an indexer?
In addition to that, the memory in the code segment has the instructions of the code of methods. This code is common for all instances.
In addition to that, there are class tables and virtual tables to describe types, methods signatures and polymorphism rules.
If the object is instantiated in a method it uses the heap memory.
If the object is instantiated in the declaration as a class member, I don't know how works .NET but it may be allocated in the data segment of the processus.
And memory is like a train where wagoons are the bytes.
Here is a pseudo-diagram of the memory.
It is not the very true reality but it may help to understand:
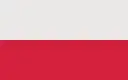
Does accessing a variable in C# class reads the entire class from memory?
C# Heap(ing) Vs Stack(ing) In .NET
A byte is the elementary unit of the memory that stores one value at a time between 0 and 255 (unsigned) or -128 and +127 (signed).
Learn the basics about C# data types' variables
Shifting Behavior for Signed Integers
A Tutorial on Data Representation
Seeing this sketch today (2021.01.28) I realize it may be mlisleading, and it is why I wrote "It is not the very true reality but it may help to understand", because in reality the code of the implementation of methods are loaded from the binary files EXE and DLLs when the process is starded and is stored is the CODE SEGMENT, as all data, static (literals) and dynamic (instances) are in the DATA SEGMENT (if things had not changed since x32 and protected mode). Methods' non-virtual tables as well as methods' virtual tables are not stored in the data segment for each instance of objects. I don't remember details but these tables is for code. Also Data of each instance of an object is a projection from its definition as well as its ancestors, in one place, one full instance.
Memory segmentation
x86 memory segmentation