Since RealityKit still doesn't have the isDoubleSided instance property that you can find in SceneKit, I offer two workarounds to bypass this limitation.
Autodesk Maya –> Solution 1
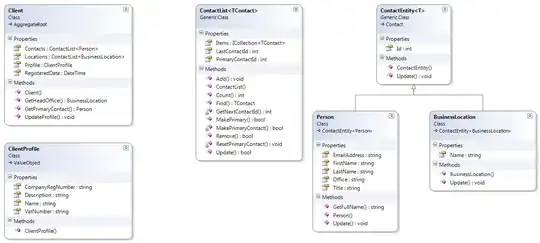
In Autodesk Maya, create two polygonal cubes, then slightly scale one of them down, then select it and apply Mesh Display > Reverse command from the Modeling
main menu set. This command reverses faces' normals 180 degrees. Export both models (first, with default normals direction, and second, with reversed normals). Here's the Python code for Maya Script Editor
:
import maya.cmds as cmds
cmds.polyCube(n='InnerCube', w=2.99, h=2.99, d=2.99)
# reversing normals of polyfaces
cmds.polyNormal(nm=0)
cmds.polyCube(n='OuterCube', w=3, h=3, d=3)
cmds.select(clear=True)
After that, import both models into the RealityKit.
RealityKit –> Solution 2

To do it programmatically in RealityKit, load original model and its duplicate into a scene, then for inner model, use faceCulling = .front
to cull front-facing polygons. Scale down the internal model slightly.
Also, you can use culling for such effects as outline border.
import UIKit
import RealityKit
class ViewController: UIViewController {
@IBOutlet var arView: ARView!
override func viewDidLoad() {
super.viewDidLoad()
let boxScene = try! Experience.loadBox()
// INNER
var innerMaterial = PhysicallyBasedMaterial()
innerMaterial.faceCulling = .front // face culling
innerMaterial.baseColor.tint = .green
let box = boxScene.steelBox?.children[0] as! ModelEntity
box.scale = [1,1,1] * 12
box.model?.materials[0] = innerMaterial
box.name = "Inner_Green"
arView.scene.anchors.append(boxScene)
// OUTER
var outerMaterial = SimpleMaterial()
let outerBox = box.clone(recursive: false)
outerBox.model?.materials[0] = outerMaterial
outerBox.scale = [1,1,1] * 12.001
outerBox.name = "Outer_White"
// boxScene.steelBox?.addChild(outerBox) // Enable it
print(boxScene)
}
}