Jest
I've had the same issue: Jest was throwing errors related to files that I didn't use, only because they were exported in a barrel file which was used by the modules I was testing.
In my case the issue was with tests on Vuex stores which started to break because of modules using the same stores (probably due to circular dependencies).
prices.test.js
// the test imports a module
import prices from '@/store/modules/prices';
prices.js
// the module imports a module from a barrel file
import { isObject } from '@/common';
index.js
// this is the imported module
export { default as isObject } from './isObject';
// this is the other module that breaks my tests, even if I'm not importing it
export { default as getPageTitle } from './getPageTitle';
getPageTitle.js
// when the module uses the store, an error is thrown
import store from '@/store/store';
I think that in my case the issue was a circular dependency, anyway to answer your question: yes, Jest imports all the modules in the barrel files even if they are not imported directly.
In my case the solution was to move the modules that were using Vuex to a separate folder and to create a separate barrel file only for those files.
Webpack
In a different moment I figured out that Webpack is doing the same thing by default. I didn't notice it on a project where modules were small and weren't importing libraries, but on a separate project I had modules importing very large libraries and I noticed that Webpack wasn't doing tree-shaking and wasn't optimizing chunks as it was supposed to do.
I discovered that Webpack imports all the libraries in the barrel file, in a similar way as Jest does. The upside is that you can stop this behavior by disabling side effects on the specific barrel files.
webpack.config.js
{
module: {
rules: [
// other rules...
{
test: [/src\/common\/index.ts/i],
sideEffects: false,
}
]
}
}
You can see the difference using Webpack Bundle Analyzer: when side effects are not turned off, all imports from one specific folder will have the same size. On the other hand, when side effects are turned off you will see a different size for different imports.
Default behavior (all imports the same size)
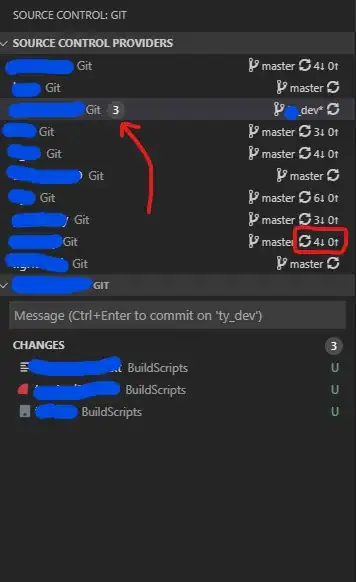
Side effects turned off (the size depends on which modules you import)
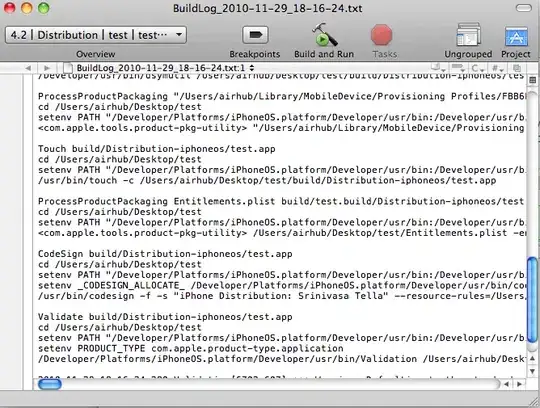
When files in the barrel files import large libraries, the improvements can be even more noticeable (tree-shaking works, chunks are optimized).
You can find more details here: https://github.com/vercel/next.js/issues/12557 and here: Webpack doesn't split a huge vendor file when modules are exported and imported using index files.